FFmpeg从packet中拆分出NAL单元的完整代码
时间: 2024-06-08 07:07:33 浏览: 151
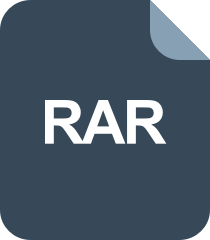
FFmpeg - 将mp4转为gif输出(代码方式)

首先需要了解一下H.264/AVC视频编码的基本结构。H.264视频编码将视频帧分为若干个片(slice),每个片又分为若干个宏块(macroblock),宏块再分为若干个子块(sub-block)。每个子块的编码数据就是一个NAL单元,也就是网络抽象层单元。
在FFmpeg中,可以通过解码器(decoder)获取到视频帧的packet,每个packet对应一个或多个NAL单元。下面是从packet中拆分出NAL单元的完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <errno.h>
#include <inttypes.h>
#include "libavcodec/avcodec.h"
#define BUFFER_SIZE 1024 * 1024
int main(int argc, char **argv)
{
if (argc < 2) {
fprintf(stderr, "Usage: %s <input_file>\n", argv[0]);
exit(1);
}
const char *input_file = argv[1];
AVCodec *codec = avcodec_find_decoder(AV_CODEC_ID_H264);
if (!codec) {
fprintf(stderr, "Codec not found\n");
exit(1);
}
AVCodecContext *context = avcodec_alloc_context3(codec);
if (!context) {
fprintf(stderr, "Could not allocate codec context\n");
exit(1);
}
if (avcodec_open2(context, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
exit(1);
}
FILE *file = fopen(input_file, "rb");
if (!file) {
fprintf(stderr, "Could not open input file '%s': %s\n", input_file, strerror(errno));
exit(1);
}
uint8_t *buffer = (uint8_t *) malloc(BUFFER_SIZE);
int buffer_size = BUFFER_SIZE;
AVPacket packet;
av_init_packet(&packet);
while (1) {
int bytes_read = fread(buffer, 1, buffer_size, file);
if (bytes_read < 0) {
fprintf(stderr, "Error while reading input file: %s\n", strerror(errno));
exit(1);
}
if (bytes_read == 0) {
break; // end of file
}
uint8_t *data = buffer;
int size = bytes_read;
while (size > 0) {
int ret = av_parser_parse2(context->parser, context, &packet.data, &packet.size, data, size, AV_NOPTS_VALUE, AV_NOPTS_VALUE, 0);
if (ret < 0) {
fprintf(stderr, "Error while parsing\n");
exit(1);
}
data += ret;
size -= ret;
if (packet.size > 0) {
printf("NAL Unit: size=%d\n", packet.size);
for (int i = 0; i < packet.size; i++) {
printf("%02x ", packet.data[i]);
}
printf("\n");
}
av_packet_unref(&packet);
}
}
free(buffer);
fclose(file);
avcodec_free_context(&context);
return 0;
}
```
这段代码的大致流程如下:
1. 使用AVCodecContext和AVCodec打开H.264解码器。
2. 打开输入文件,并分配输入缓冲区。
3. 循环读取输入文件,每次读取一定数量的数据到缓冲区中。
4. 使用解码器的parser从缓冲区中解析出NAL单元,并输出NAL单元的大小和数据。
5. 释放解码器返回的AVPacket。
注意,这段代码只是演示了如何从H.264视频中拆分出NAL单元,并没有进行任何解码操作。如果需要对视频进行解码,还需要使用解码器的API进行解码。
阅读全文
相关推荐
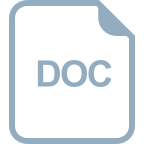
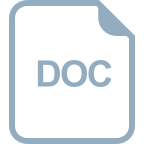

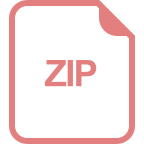
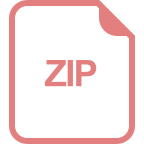
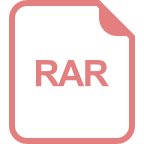
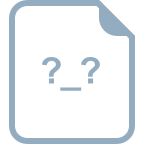
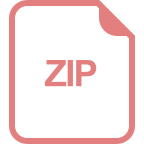
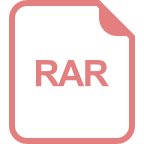
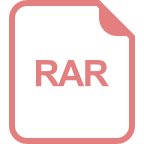
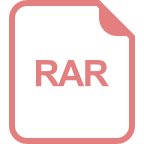
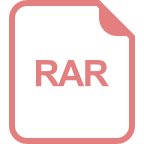
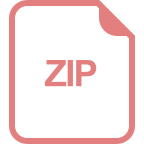
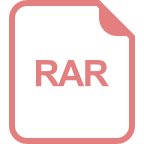
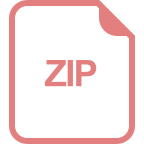