FFmpeg中从packet解析出h264的NAL单元完整代码
时间: 2024-06-08 08:07:32 浏览: 170
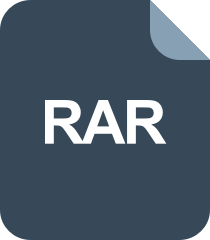
从ffmpeg中提取出来的h264解码源代码

下面是从 FFmpeg 的 packet 中解析出 H.264 的 NAL 单元的完整代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <libavcodec/avcodec.h>
#include <libavutil/pixdesc.h>
int main(int argc, char *argv[])
{
AVPacket packet;
av_init_packet(&packet);
AVCodec *codec = avcodec_find_decoder(AV_CODEC_ID_H264);
if (!codec) {
fprintf(stderr, "Codec not found\n");
return -1;
}
AVCodecParserContext *parser = av_parser_init(codec->id);
if (!parser) {
fprintf(stderr, "Parser not found\n");
return -1;
}
AVCodecContext *codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
fprintf(stderr, "Could not allocate codec context\n");
return -1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
fprintf(stderr, "Could not open codec\n");
return -1;
}
int ret = av_parser_parse2(parser, codec_ctx, &packet.data, &packet.size,
NULL, 0, AV_NOPTS_VALUE, AV_NOPTS_VALUE, 0);
if (ret < 0) {
fprintf(stderr, "Error while parsing\n");
return -1;
}
uint8_t *data = packet.data;
int size = packet.size;
while (size > 0) {
int len = av_parser_parse2(parser, codec_ctx, &data, &size,
NULL, 0, AV_NOPTS_VALUE, AV_NOPTS_VALUE, 0);
if (len < 0) {
fprintf(stderr, "Error while parsing\n");
return -1;
}
if (len > 0) {
printf("NAL unit size: %d\n", len);
printf("NAL unit type: %d\n", parser->pict_type);
}
}
av_parser_close(parser);
avcodec_free_context(&codec_ctx);
return 0;
}
```
这段代码使用了 FFmpeg 的 AVCodecParserContext 结构体来解析 H.264 的 NAL 单元。它首先初始化了一个 AVPacket 结构体,并将其传递给 av_parser_parse2 函数来解析 packet 中的数据。然后,它使用一个循环来迭代每个 NAL 单元,并打印出单元的大小和类型。
请注意,这段代码仅演示了如何从 packet 中解析 H.264 的 NAL 单元。要将这些单元解码为图像,您需要使用 FFmpeg 的解码器。
阅读全文
相关推荐




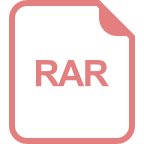
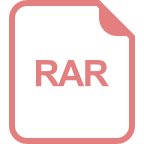
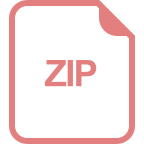
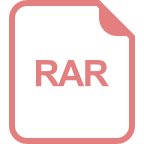
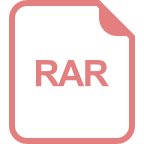
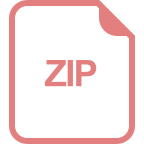
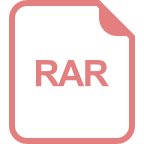
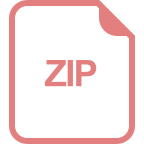
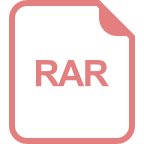
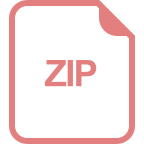
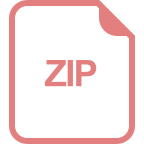
