data, addr = sock_udp.recvfrom(1024) print '[UDP] Total Data len recv ' + str(len(data)) id = struct.unpack('>H', data[0:2])[0] query = data[12:] data = dw(id) # id data += dw(0x85a0) # flags data += dw(1) # questions data += dw(0x52) # answers data += dw(0) # authoritative data += dw(0) # additional # Add the question back - we're just hardcoding it data += ('\x03125\x018\x018\x018\x07in-addr\x04arpa\x00' + '\x00\x0c' + # type = 'PTR' '\x00\x01') # cls = 'IN' # Add the first answer data += ('\xc0\x0c' + # ptr to the name '\x00\x0c' + # type = 'PTR' '\x00\x01' + # cls = 'IN' '\x00\x00\x00\x3d' + # ttl '\x04\x00' + # size of this resource record '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x3e' + 'Z'*62 + '\x0e' + 'Z'*14 + '\x00') # Add the next answer, which is written out in full data += ('\xc0\x0c' + # ptr to the name '\x00\x0c' + # type = 'PTR' '\x00\x01' + # cls = 'IN' '\x00\x00\x00\x3d' + # ttl '\x00\x26' + # size of this resource record '\x08DCBBEEEE\x04DDDD\x08CCCCCCCC\x04AAAA\x04BBBB\x03com\x00')
时间: 2024-04-27 10:24:11 浏览: 11
这段代码实现了一个 DNS 服务器的响应报文的构造过程。首先,使用 UDP 协议接收来自客户端的 DNS 查询报文(不超过 1024 字节),其中包含了查询的 domain 名称,然后解析出查询的 id 和问题部分的数据。接着,根据 DNS 协议的规定,构造 DNS 响应报文,并将其打包成二进制数据。具体来说,响应报文的格式如下:
1. 16 位的 id,与查询报文的 id 相同。
2. 16 位的 flags,表示响应报文的状态信息。
3. 16 位的 questions,表示查询的问题数,这里为 1。
4. 16 位的 answers,表示响应的回答数,这里为 1。
5. 16 位的 authoritative,表示授权回答数,这里为 0。
6. 16 位的 additional,表示附加回答数,这里为 0。
接着,构造响应报文中的 question 部分,这里是一个固定的反向 DNS 查询报文,即查询 IP 地址对应的域名。然后,构造响应报文中的 answer 部分,包含了两个 PTR 类型的回答记录,分别是查询 IP 地址对应的两个域名的反向映射结果。其中,第一个 PTR 记录的数据部分由 16 个 62 字节的字符串和一个 14 字节的字符串组成,第二个 PTR 记录的数据部分是一个完整的 PTR 记录,它将查询 IP 地址映射到一个域名。最后,将构造好的 DNS 响应报文通过 UDP 协议发送给客户端。
相关问题
优化并改编以下代码,使其和原来有部分出入但实现效果相同: 1. import socket 2. 3. 4. def receive(): 5. # 创建套接字 6. udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) 7. 8. # 准备数据9. file_name = input("Please input the save file name:") 10. 11. # 发送数据 12. ip = input("Please input the sender's ipv4 address:") 13. udp_socket.sendto(file_name.encode('gbk'), (ip, 7788)) 14. 15. # 接收数据 16. recv_data = udp_socket.recvfrom(1024) 17. file_data = recv_data[0] 18. with open(file_name, 'wb') as f: 19. f.write(file_data) 20. print("Receive successfully!") 21. # 关闭套接字 22. udp_socket.close() 23. 24. 25.def send(): 26. # 创建套接字 27. udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) 28. 29. # 绑定本地信息 30. localaddr = ('', 7788) 31. udp_socket.bind(localaddr) 32. 33. # 接收数据 34. while True: 35. recv_data = udp_socket.recvfrom(1024) 36. recv_msg = recv_data[0] 37. send_addr = recv_data[1] 38. print("%s:%s" % (str(send_addr), recv_msg.decode('gbk'))) 39. 40. # 读取文件并传输文件 41. with open(recv_msg.decode('gbk'), 'rb') as f: 42. file_data = f.read() 43. udp_socket.sendto(file_data, send_addr) 44. 45. print("Send successfully!") 46. break 47. 48. # 关闭套接字 49. udp_socket.close() 50. 51. 52.if name == 'main': 3553. while True: 54. answer = input("This is a simple program relying on the Udp protocol, \nif you want to send the file," 55. "please input 1,\n if you want to receive th e file, please input 2, \n if you want exit, " 56. "please input 0:\n") 57. if answer == '0': 58. break 59. if answer == '1': 60. send() 61. if answer == '2': 62. receive()
以下是优化并改编后的代码,改动主要是将 receive() 和 send() 函数中的 input() 改为函数参数接收:
```
import socket
def receive(file_name, ip):
# 创建套接字
udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 发送数据
udp_socket.sendto(file_name.encode('gbk'), (ip, 7788))
# 接收数据
recv_data = udp_socket.recvfrom(1024)
file_data = recv_data[0]
with open(file_name, 'wb') as f:
f.write(file_data)
print("Receive successfully!")
# 关闭套接字
udp_socket.close()
def send(file_path):
# 创建套接字
udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 绑定本地信息
localaddr = ('', 7788)
udp_socket.bind(localaddr)
# 接收数据
while True:
recv_data = udp_socket.recvfrom(1024)
recv_msg = recv_data[0]
send_addr = recv_data[1]
print("%s:%s" % (str(send_addr), recv_msg.decode('gbk')))
# 读取文件并传输文件
with open(file_path, 'rb') as f:
file_data = f.read()
udp_socket.sendto(file_data, send_addr)
print("Send successfully!")
break
# 关闭套接字
udp_socket.close()
if __name__ == '__main__':
while True:
answer = input("This is a simple program relying on the Udp protocol, \nif you want to send the file, please input 1,\nif you want to receive the file, please input 2, \nif you want exit, please input 0:\n")
if answer == '0':
break
if answer == '1':
file_path = input("Please input the file path to send:")
send(file_path)
if answer == '2':
file_name = input("Please input the save file name:")
ip = input("Please input the sender's ipv4 address:")
receive(file_name, ip)
```
改编后的代码中,receive() 和 send() 函数分别接收一个参数,即文件名或文件路径,而不是通过 input() 函数从命令行读取。这样可以使代码更加模块化和可重用。同时,代码中的注释也进行了修改和补充,以提高代码可读性和易懂性。
用python为下面代码实现GUI,要求:仅实现类似聊天框功能即可,ip地址和port在代码中写入不能更改。代码:import time import socket #import tk def main(): ip = "172.20.10.13" port = 8888 other_addr = (ip, port) byte = 1024 udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM) while True: send_data = input("发送给客户端:").encode("utf-8") udp_socket.sendto(send_data, other_addr) #输入数据为空退出,否则进入接收状态 if send_data: rev_data, other_addr = udp_socket.recvfrom(byte) localTime = time.asctime(time.localtime(time.time())) print(localTime,"收到来自%s的信息: %s" % (other_addr, rev_data.decode("utf-8"))) else: break udp_socket.close() if __name__ == '__main__': main()
以下是使用Python tkinter模块实现的GUI代码,实现了类似聊天框的功能:
```python
import time
import socket
import tkinter as tk
# 创建GUI窗口
root = tk.Tk()
root.title('UDP聊天')
root.geometry('400x400')
# 创建文本框和滚动条
text = tk.Text(root)
text.pack(side=tk.LEFT, fill=tk.BOTH, expand=True)
scrollbar = tk.Scrollbar(root)
scrollbar.pack(side=tk.RIGHT, fill=tk.Y)
# 将文本框和滚动条关联
text.config(yscrollcommand=scrollbar.set)
scrollbar.config(command=text.yview)
# 创建输入框和发送按钮
input_frame = tk.Frame(root)
input_frame.pack(side=tk.BOTTOM, fill=tk.X)
input_entry = tk.Entry(input_frame)
input_entry.pack(side=tk.LEFT, fill=tk.X, expand=True)
send_button = tk.Button(input_frame, text='发送')
send_button.pack(side=tk.RIGHT)
# 设置UDP通信参数
ip = "172.20.10.13"
port = 8888
other_addr = (ip, port)
byte = 1024
udp_socket = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
# 定义发送函数
def send_msg():
send_data = input_entry.get().encode("utf-8")
udp_socket.sendto(send_data, other_addr)
localTime = time.asctime(time.localtime(time.time()))
text.insert(tk.END, localTime + ' 发送给客户端: ' + input_entry.get() + '\n')
input_entry.delete(0, tk.END)
# 定义接收函数
def recv_msg():
rev_data, other_addr = udp_socket.recvfrom(byte)
localTime = time.asctime(time.localtime(time.time()))
text.insert(tk.END, localTime + ' 收到来自%s的信息: %s \n' % (other_addr, rev_data.decode("utf-8")))
# 设置窗口关闭函数
def close_window():
udp_socket.close()
root.destroy()
# 绑定发送按钮和发送函数
send_button.config(command=send_msg)
# 接收消息并插入到文本框中
root.after(100, recv_msg)
# 启动GUI程序
root.protocol('WM_DELETE_WINDOW', close_window)
root.mainloop()
```
运行该代码会弹出一个窗口,可以在输入框中输入消息并点击发送按钮发送消息,接收到的消息会显示在文本框中。同时,程序会持续接收UDP消息并自动更新到文本框中。点击窗口右上角的关闭按钮会关闭UDP连接并退出程序。
相关推荐
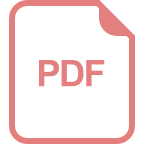
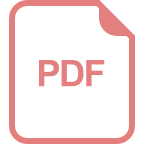
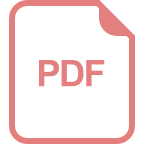












