两条直线相交点求法c++
时间: 2023-07-04 17:20:50 浏览: 232
可以使用解方程的方法来求两条直线的交点,具体步骤如下:
1.将两条直线表示为一般式方程:Ax + By = C。
2.将两条直线的方程转化为矩阵形式,即:
```
| A1 B1 | | x | | C1 |
| | * | | = | |
| A2 B2 | | y | | C2 |
```
其中,A1、B1、C1表示第一条直线的系数,A2、B2、C2表示第二条直线的系数。
3.求解上述矩阵方程,即可得到交点的坐标。
以下是使用C++代码实现上述方法的示例:
```c++
#include <iostream>
using namespace std;
struct Point {
double x;
double y;
};
bool getIntersection(Point p1, Point p2, Point p3, Point p4, Point& intersection) {
double d = (p1.x - p2.x) * (p3.y - p4.y) - (p1.y - p2.y) * (p3.x - p4.x);
if (d == 0) { // 两条直线平行
return false;
}
double x = ((p1.x * p2.y - p1.y * p2.x) * (p3.x - p4.x) - (p1.x - p2.x) * (p3.x * p4.y - p3.y * p4.x)) / d;
double y = ((p1.x * p2.y - p1.y * p2.x) * (p3.y - p4.y) - (p1.y - p2.y) * (p3.x * p4.y - p3.y * p4.x)) / d;
intersection.x = x;
intersection.y = y;
return true;
}
int main() {
Point p1 = {1, 2};
Point p2 = {3, 4};
Point p3 = {5, 6};
Point p4 = {7, 8};
Point intersection;
if (getIntersection(p1, p2, p3, p4, intersection)) {
cout << "Intersection: (" << intersection.x << ", " << intersection.y << ")" << endl;
} else {
cout << "Parallel lines" << endl;
}
return 0;
}
```
注意,如果两条直线平行,则无交点。因此,需要在函数中对此情况进行判断并返回相应的结果。
阅读全文
相关推荐
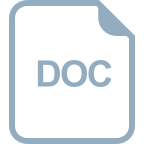
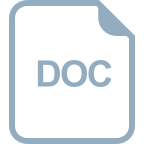
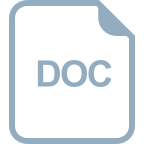
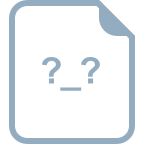
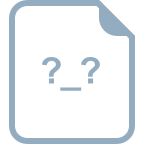

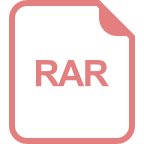
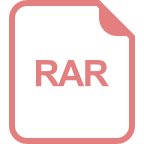
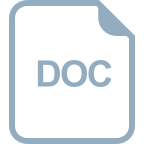









