Python求固定点到固定点的最短路
时间: 2023-10-11 12:17:52 浏览: 158
你可以使用Dijkstra算法来解决这个问题。
Dijkstra算法是一种贪心算法,用于求解带权有向图中单源最短路径问题。假设我们要从起点s到终点t,我们可以在图中运行Dijkstra算法,找到从s到t的最短路径。
下面是一个Python实现的Dijkstra算法,用于求解固定点到固定点的最短路:
```python
import heapq
def dijkstra(graph, start, end):
distances = {vertex: float('infinity') for vertex in graph}
distances[start] = 0
pq = [(0, start)]
while pq:
current_distance, current_vertex = heapq.heappop(pq)
if current_vertex == end:
return current_distance
for neighbor, weight in graph[current_vertex].items():
distance = current_distance + weight
if distance < distances[neighbor]:
distances[neighbor] = distance
heapq.heappush(pq, (distance, neighbor))
return float('infinity')
```
在这个代码中,我们假设输入的是一个字典类型的graph,其中键是所有节点的名称,值是一个字典,其中键是所有与该节点相邻的节点,值是从该节点到相邻节点的权重。
start和end是我们要找到最短路径的起点和终点。
在算法中,我们首先初始化所有节点的距离为无穷大,然后将起点的距离设置为0。我们使用一个优先队列pq来保存每个节点的距离和节点名称。在每一轮迭代中,我们从pq中弹出当前距离最小的节点,并更新所有相邻节点的距离。如果我们找到了终点,就返回到该节点的距离。
使用这个算法,你可以找到固定点到固定点的最短路。
阅读全文
相关推荐
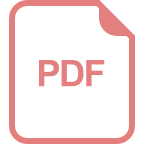
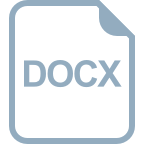
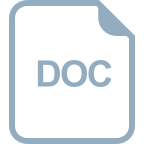
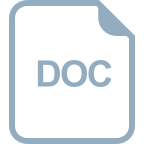
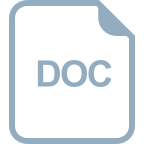
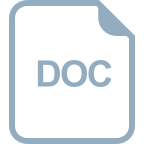
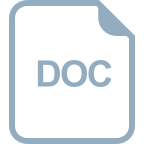
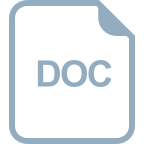
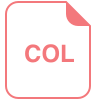
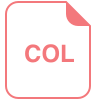
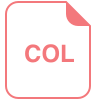
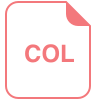
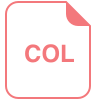
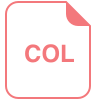
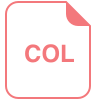