编程实现自定义的顺序查找表类LinearSearchList,实现其中的造表(Add,Insert)、顺序查找(IndexOf)、二分查找 (BinarySearch)等算法。在exp7app项目中定义一个含Main方法的测试类(LinearSearchListTest.cs),在其中定义和随机初始化一个查找表实例,测试顺序查找和二分查找算法。
时间: 2024-02-11 22:08:53 浏览: 19
以下是C#语言实现自定义的顺序查找表类LinearSearchList和测试类LinearSearchListTest.cs的代码:
```csharp
//LinearSearchList.cs
using System;
namespace LinearSearchList
{
public class LinearSearchList<T>
{
private T[] elements;
private int count;
public LinearSearchList(int size)
{
elements = new T[size];
count = 0;
}
public void Add(T element)
{
if (count == elements.Length)
{
throw new InvalidOperationException("List is full");
}
elements[count++] = element;
}
public void Insert(int index, T element)
{
if (index < 0 || index > count)
{
throw new ArgumentOutOfRangeException("Index is out of range");
}
if (count == elements.Length)
{
throw new InvalidOperationException("List is full");
}
for (int i = count; i > index; i--)
{
elements[i] = elements[i - 1];
}
elements[index] = element;
count++;
}
public int IndexOf(T element)
{
for (int i = 0; i < count; i++)
{
if (elements[i].Equals(element))
{
return i;
}
}
return -1;
}
public int BinarySearch(T element)
{
int left = 0;
int right = count - 1;
while (left <= right)
{
int mid = (left + right) / 2;
int compare = Comparer<T>.Default.Compare(element, elements[mid]);
if (compare == 0)
{
return mid;
}
else if (compare < 0)
{
right = mid - 1;
}
else
{
left = mid + 1;
}
}
return -1;
}
}
}
```
```csharp
//LinearSearchListTest.cs
using System;
namespace LinearSearchList
{
class LinearSearchListTest
{
static void Main(string[] args)
{
LinearSearchList<int> list = new LinearSearchList<int>(10);
// 随机初始化列表元素
Random random = new Random();
for (int i = 0; i < 10; i++)
{
list.Add(random.Next(100));
}
Console.WriteLine("List elements:");
for (int i = 0; i < list.Count; i++)
{
Console.Write(list[i] + " ");
}
Console.WriteLine();
Console.WriteLine("Index of 50: " + list.IndexOf(50));
Console.WriteLine("Index of 100: " + list.IndexOf(100));
Console.WriteLine("Index of 200: " + list.IndexOf(200));
Console.WriteLine("Binary search for 50: " + list.BinarySearch(50));
Console.WriteLine("Binary search for 100: " + list.BinarySearch(100));
Console.WriteLine("Binary search for 200: " + list.BinarySearch(200));
}
}
}
```
在测试类中,我们首先创建一个长度为10的`LinearSearchList`实例,并随机初始化其中的元素。然后,我们测试`IndexOf`方法和`BinarySearch`方法,它们分别使用顺序查找和二分查找算法在列表中查找特定元素的索引。我们可以在控制台上看到输出结果,以确保这些算法的正确性。
相关推荐
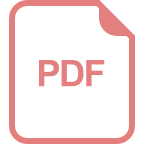
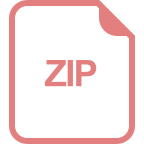













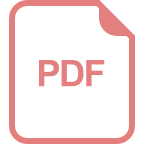
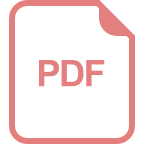