写一段java代码,【问题描述】 定义一个Student类,包含姓名(name)、身高(height)、体重(weight),分数(score)以及speak()方法,该方法的功能是,输出自己的相关信息。 Student类实现Comparable接口,实现比较两个Student对象的大小,比较规则是:身高和体重各占30%权重,分数占40%权重,以综合计算三项权重和之后的值作为判断对象大小的依据。 最后,定义一个测试类,生成一个数组,该数组有6个元素,每个元素类型是Student,调用Arrays.sort方法对该数组排序。 按照以上描述,以及输出样例的结果,完成程序代码的填写。 【输入形式】 无 【输出形式】 数组排序前以及排序后的结果。 【样例输入】 无 【样例输出】 array sort before: I am zhangsan,my height 170.0,my weight 110.0,my score 95.0 I am lisi,my height 168.0,my weight 120.0,my score 75.0 I am wangwu,my height 165.0,my weight 115.0,my score 88.0 I am zhaoliu,my height 172.0,my weight 121.0,my score 90.0 I am zhouqi,my height 160.0,my weight 100.0,my score 85.0 I am zhengba,my height 166.0,my weight 119.0,my score 70.0 array sort after: Student [name=zhouqi,height=160.0,weight=100.0,score=85.0] Student [name=zhengba,height=166.0,weight=119.0,score=70.0] Student [name=lisi,height=168.0,weight=120.0,score=75.0] Student [name=wangwu,height=165.0,weight=115.0,score=88.0] Student [name=zhangsan,height=170.0,weight=110.0,score=95.0] Student [name=zhaoliu,height=172.0,weight=121.0,score=90.0]
时间: 2024-03-15 20:44:46 浏览: 55
```
import java.util.Arrays;
public class Student implements Comparable<Student>{
private String name;
private double height;
private double weight;
private double score;
public Student(String name, double height, double weight, double score) {
this.name = name;
this.height = height;
this.weight = weight;
this.score = score;
}
public void speak() {
System.out.println("I am " + name + ",my height " + height + ",my weight " + weight + ",my score " + score);
}
@Override
public int compareTo(Student o) {
double weightSum = height * 0.3 + weight * 0.3 + score * 0.4;
double oWeightSum = o.height * 0.3 + o.weight * 0.3 + o.score * 0.4;
if(weightSum > oWeightSum) {
return 1;
} else if(weightSum < oWeightSum) {
return -1;
} else {
return 0;
}
}
public static void main(String[] args) {
Student[] students = new Student[6];
students[0] = new Student("zhangsan", 170.0, 110.0, 95.0);
students[1] = new Student("lisi", 168.0, 120.0, 75.0);
students[2] = new Student("wangwu", 165.0, 115.0, 88.0);
students[3] = new Student("zhaoliu", 172.0, 121.0, 90.0);
students[4] = new Student("zhouqi", 160.0, 100.0, 85.0);
students[5] = new Student("zhengba", 166.0, 119.0, 70.0);
System.out.println("array sort before:");
for(Student student : students) {
student.speak();
}
Arrays.sort(students);
System.out.println("\narray sort after:");
for(Student student : students) {
System.out.println(student.toString());
}
}
@Override
public String toString() {
return "Student [name=" + name + ",height=" + height + ",weight=" + weight + ",score=" + score + "]";
}
}
```
相关推荐
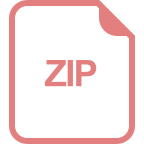
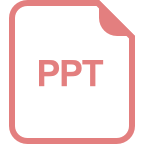
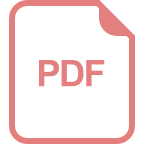















