python 词频统计
时间: 2023-09-07 07:17:31 浏览: 87
可以使用Python中的collections模块来进行词频统计。以下是一个简单的示例代码:
```python
import collections
# 读取文件
with open('example.txt', 'r') as f:
text = f.read()
# 分词
words = text.split()
# 统计词频
word_counts = collections.Counter(words)
# 输出前10个出现频率最高的词
for word, count in word_counts.most_common(10):
print(word, count)
```
其中,example.txt是待分析的文本文件。上述代码将文本文件读入内存后,使用split()方法将文本分为单个单词的列表。然后,使用Counter函数统计每个单词在列表中出现的次数,最后输出出现频率最高的前10个单词及其出现次数。
相关问题
python词频统计
您可以使用Python中的collections库来实现词频统计。以下是一个简单的例子:
```python
import collections
text = "Python is an interpreted high-level programming language for general-purpose programming. Created by Guido van Rossum and first released in 1991, Python has a design philosophy that emphasizes code readability, notably using significant whitespace. It provides constructs that enable clear programming on both small and large scales."
words = text.lower().split() # 将文本转换为小写并按空格分割成单词列表
word_counts = collections.Counter(words) # 统计单词出现的次数
print(word_counts)
```
输出结果为:
```
Counter({'programming': 2, 'python': 2, 'and': 2, 'is': 1, 'an': 1, 'interpreted': 1, 'high-level': 1, 'language': 1, 'for': 1, 'general-purpose': 1, 'created': 1, 'by': 1, 'guido': 1, 'van': 1, 'rossum': 1, 'first': 1, 'released': 1, 'in': 1, '1991,': 1, 'has': 1, 'a': 1, 'design': 1, 'philosophy': 1, 'that': 1, 'emphasizes': 1, 'code': 1, 'readability,': 1, 'notably': 1, 'using': 1, 'significant': 1, 'whitespace.': 1, 'it': 1, 'provides': 1, 'constructs': 1, 'enable': 1, 'clear': 1, 'on': 1, 'both': 1, 'small': 1, 'large': 1, 'scales.': 1})
```
其中,Counter对象是一个字典,包含每个单词出现的次数。
Python词频统计
Python词频统计是指使用Python编程语言来统计一段文本中各个单词出现的频率。通过词频统计,我们可以了解到文本中哪些单词出现的次数较多,从而对文本进行分析和处理。
在Python中,可以使用字典(dictionary)来实现词频统计。具体步骤如下:
1. 将文本读入到Python程序中,可以使用文件读取函数或者直接将文本赋值给一个字符串变量。
2. 对文本进行预处理,例如去除标点符号、转换为小写等操作,以便统计的准确性。
3. 将文本按照空格或其他分隔符进行分割,得到单词列表。
4. 遍历单词列表,使用字典记录每个单词出现的次数。如果单词已经在字典中,则将其对应的值加1;如果单词不在字典中,则将其添加到字典,并将值初始化为1。
5. 根据词频进行排序,可以使用sorted函数或者其他排序方法对字典按照值进行排序。
6. 输出排序后的结果,可以选择输出前几个高频词或者输出所有的词频统计结果。
下面是一个简单的Python代码示例:
```python
text = "This is a sample text for word frequency analysis. This is just a sample."
word_list = text.lower().split()
word_count = {}
for word in word_list:
if word in word_count:
word_count[word] += 1
else:
word_count[word] = 1
sorted_word_count = sorted(word_count.items(), key=lambda x: x, reverse=True)
for word, count in sorted_word_count:
print(word, count)
```
这段代码会输出每个单词及其出现的次数,按照词频从高到低排序。
阅读全文
相关推荐
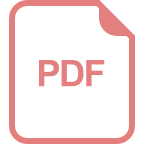









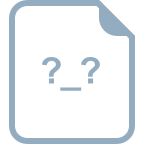
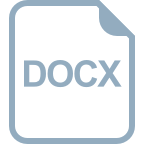