carla中为什么设置了触发器,但是NPC经过该触发器并没有销毁
时间: 2023-12-04 12:27:15 浏览: 127
在CARLA中,设置触发器后,NPC并不会自动销毁,触发器只是用来检测NPC是否进入或离开触发器的范围。如果你希望NPC在触发器内销毁,可以在触发器的事件中添加销毁NPC的代码。例如,在Python中,你可以在触发器的回调函数中调用`destroy()`函数来销毁NPC。具体实现方式如下:
```python
def on_collision(other_actor):
if isinstance(other_actor, carla.WheeledVehicle):
# 如果碰到的是NPC车辆,则销毁该车辆
other_actor.destroy()
```
在这个例子中,我们检测到碰撞发生时,判断碰撞对象是否为NPC车辆,如果是则调用`destroy()`函数销毁该车辆。你可以根据实际需求修改回调函数的实现方式。
相关问题
carla中在NPC的终点设置一个触发器,使得该辆NPC一旦到达终点就会自动销毁,并在此时自动在起点生成该辆NPC
在Carla中,可以通过设置与终点位置关联的触发器来实现NPC到达终点后自动销毁并重新生成NPC的功能。具体步骤如下:
1. 在Carla中,使用Python API创建NPC车辆,并将其设置到起点位置。
2. 在Carla中,使用Python API创建一个Box型碰撞体,并将其设置到终点位置。
3. 监听碰撞事件,当NPC车辆进入碰撞体时,销毁当前NPC,并重新创建一个新的NPC并将其设置到起点位置。
下面是一个示例代码:
```python
import carla
class NPCController:
def __init__(self, world, start_location, end_location):
self.world = world
self.start_location = start_location
self.end_location = end_location
self.current_npc = None
self.collision_sensor = None
def spawn_npc(self):
blueprint_library = self.world.get_blueprint_library()
npc_bp = blueprint_library.filter('vehicle.*')[0]
spawn_transform = carla.Transform(self.start_location)
self.current_npc = self.world.spawn_actor(npc_bp, spawn_transform)
self.current_npc.set_target_location(carla.Location(self.end_location))
# 添加碰撞检测器
collision_bp = blueprint_library.find('sensor.other.collision')
self.collision_sensor = self.world.spawn_actor(collision_bp, carla.Transform(), attach_to=self.current_npc)
self.collision_sensor.listen(lambda event: self.on_collision(event))
def on_collision(self, event):
if event.other_actor.id == self.current_npc.id:
self.current_npc.destroy()
self.spawn_npc()
if __name__ == '__main__':
client = carla.Client('localhost', 2000)
client.set_timeout(10.0)
world = client.get_world()
start_location = [0, 0, 0] # 起点位置
end_location = [50, 50, 0] # 终点位置
controller = NPCController(world, start_location, end_location)
controller.spawn_npc()
while True:
continue
```
在上面的代码中,我们创建了一个名为NPCController的类,它包含了spawn_npc()和on_collision()两个方法。在spawn_npc()方法中,我们使用Carla的Python API创建NPC车辆,并将其设置到起点位置,然后将NPC车辆的目标位置设置为终点位置。同时,我们还添加了一个碰撞检测器,并将其附加到NPC车辆上。
在on_collision()方法中,我们监听碰撞事件,并检测碰撞的是否是当前的NPC车辆。如果是当前的NPC车辆,我们就销毁它,并重新生成一个新的NPC车辆并将其设置到起点位置。
最后,在主函数中,我们创建了一个NPCController对象,并调用spawn_npc()方法生成第一辆NPC车辆。同时,我们还使用了一个无限循环来保持程序的运行。
if isinstance(other_actor, carla.Vehicle): destroy_npc_vehicle(NPC) NPC = generate_npc_vehicle()这里的other_actor和carla.vehicle分别表示什么将这段代码改为如果和触发器重叠的是npc,则销毁该辆npc
可以将代码修改为以下形式:
```python
# 触发器事件
def on_trigger_begin_overlap(other_actor):
global NPC # 声明变量为全局变量,便于在函数中进行修改
if isinstance(other_actor, carla.Vehicle) and other_actor.attributes.get('role_name') == 'npc': # 如果与触发器重叠的是NPC
destroy_npc_vehicle(NPC) # 销毁NPC
NPC = generate_npc_vehicle() # 生成新的NPC
```
这样修改后,当与触发器重叠的是NPC时,NPC会被销毁。注意需要在函数外定义NPC变量,并在函数中使用`global`关键字声明为全局变量,以便在函数中进行修改。另外,由于NPC车辆可能会设置`role_name`属性为`npc`,因此我们加入了一个判断条件,以确保`other_actor`是一个NPC车辆。
阅读全文
相关推荐
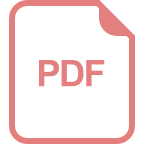
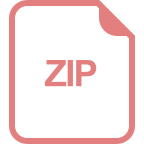








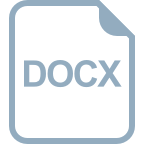
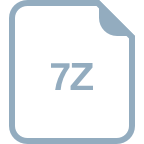