import java.util.*; public class HelloWorld { public static void main(String[] args) { System.out.println("Hello world!"); } }import java.awt.Color; import java.awt.Dimension; import java.awt.Graphics; import java.awt.Point; import java.util.ArrayList; import java.util.List; import javax.swing.JFrame; import javax.swing.JPanel; public class HeartAnimation extends JPanel implements Runnable { private List<Point> heartPoints; private int size; private Color color; private int x; private int y; public HeartAnimation(int size, Color color, int x, int y) { this.size = size; this.color = color; this.x = x; this.y = y; this.setPreferredSize(new Dimension(size, size)); this.heartPoints = new ArrayList<>(); this.createHeart(); } private void createHeart() { for (double t = 0; t <= Math.PI * 2; t += 0.01) { int x = (int) (16 * Math.pow(Math.sin(t), 3)); int y = (int) (-13 * Math.cos(t) + 5 * Math.cos(2 * t) + 2 * Math.cos(3 * t) + Math.cos(4 * t)); Point point = new Point(x * 10, y * 10); this.heartPoints.add(point); } } @Override public void paintComponent(Graphics g) { super.paintComponent(g); g.setColor(this.color); for (Point point : this.heartPoints) { int x = point.x + this.x; int y = point.y + this.y; g.drawLine(x, y, x, y); } } @Override public void run() { while (true) { try { Thread.sleep(10); this.x += 1; this.y += 1; this.repaint(); } catch (InterruptedException e) { e.printStackTrace(); } } } public static void main(String[] args) { JFrame frame = new JFrame(); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); HeartAnimation heartAnimation = new HeartAnimation(400, Color.RED, 100, 100); frame.add(heartAnimation); frame.pack(); frame.setVisible(true); Thread thread = new Thread(heartAnimation); thread.start(); } }帮我检查一下这个代码为什么无法运行
时间: 2024-01-02 07:04:57 浏览: 125
这份代码存在两个类的定义,需要将第二个类 `HeartAnimation` 的代码复制到第一个类 `HelloWorld` 的后面并将其删除。此外,在 `main` 方法中启动线程时,应该使用 `SwingUtilities.invokeLater()` 方法以确保在事件分派线程中启动线程,代码如下:
```
SwingUtilities.invokeLater(new Runnable() {
public void run() {
Thread thread = new Thread(heartAnimation);
thread.start();
}
});
```
修改后的代码如下:
```
import java.util.*;
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.util.ArrayList;
import java.util.List;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.SwingUtilities;
public class HelloWorld extends JPanel implements Runnable {
private List<Point> heartPoints;
private int size;
private Color color;
private int x;
private int y;
public HelloWorld(int size, Color color, int x, int y) {
this.size = size;
this.color = color;
this.x = x;
this.y = y;
this.setPreferredSize(new Dimension(size, size));
this.heartPoints = new ArrayList<>();
this.createHeart();
}
private void createHeart() {
for (double t = 0; t <= Math.PI * 2; t += 0.01) {
int x = (int) (16 * Math.pow(Math.sin(t), 3));
int y = (int) (-13 * Math.cos(t) + 5 * Math.cos(2 * t) + 2 * Math.cos(3 * t) + Math.cos(4 * t));
Point point = new Point(x * 10, y * 10);
this.heartPoints.add(point);
}
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
g.setColor(this.color);
for (Point point : this.heartPoints) {
int x = point.x + this.x;
int y = point.y + this.y;
g.drawLine(x, y, x, y);
}
}
@Override
public void run() {
while (true) {
try {
Thread.sleep(10);
this.x += 1;
this.y += 1;
this.repaint();
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
public static void main(String[] args) {
JFrame frame = new JFrame();
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
HelloWorld heartAnimation = new HelloWorld(400, Color.RED, 100, 100);
frame.add(heartAnimation);
frame.pack();
frame.setVisible(true);
SwingUtilities.invokeLater(new Runnable() {
public void run() {
Thread thread = new Thread(heartAnimation);
thread.start();
}
});
}
}
```
现在代码就可以正常运行了。
阅读全文
相关推荐
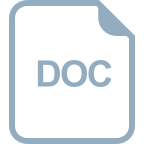
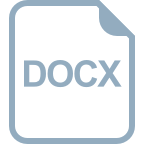
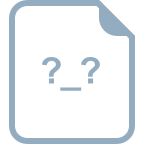








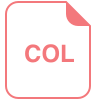
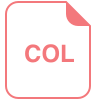





