写一个输入图像数据集的卷积GRU的pytorch-cpu代码
时间: 2023-06-09 11:04:19 浏览: 117
好的,以下是一个输入图像数据集的卷积GRU的PyTorch-CPU代码示例:
```python
import torch.nn as nn
class ConvGRU(nn.Module):
def __init__(self, input_dim, hidden_dim, kernel_size, num_layers):
super(ConvGRU, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.kernel_size = kernel_size
self.num_layers = num_layers
padding = kernel_size // 2
# Create list of convolutional GRU layers
self.conv_gru_layers = nn.ModuleList()
for i in range(self.num_layers):
input_dim = self.input_dim if i == 0 else self.hidden_dim
conv_gru_layer = ConvGRULayer(input_dim, self.hidden_dim, self.kernel_size, padding)
self.conv_gru_layers.append(conv_gru_layer)
def forward(self, x, hidden_state=None):
current_hidden_state = hidden_state
# Iterate through layers
for layer_idx in range(self.num_layers):
conv_gru_layer = self.conv_gru_layers[layer_idx]
conv_input = x if layer_idx == 0 else current_hidden_state
# Run convolutional GRU layer
layer_hidden_state = conv_gru_layer(conv_input, current_hidden_state)
if current_hidden_state is None:
current_hidden_state = layer_hidden_state
else:
current_hidden_state = current_hidden_state + layer_hidden_state
return current_hidden_state
class ConvGRULayer(nn.Module):
def __init__(self, input_dim, hidden_dim, kernel_size, padding):
super(ConvGRULayer, self).__init__()
self.input_dim = input_dim
self.hidden_dim = hidden_dim
self.kernel_size = kernel_size
self.padding = padding
# Convolutional layers to compute gates
self.conv_gates = nn.Conv2d(input_dim + hidden_dim, 2 * hidden_dim, kernel_size, padding)
# Convolutional layer to compute candidate hidden state
self.conv_candidate = nn.Conv2d(input_dim + hidden_dim, hidden_dim, kernel_size, padding)
def forward(self, x, hidden_state=None):
if hidden_state is None:
hidden_state = torch.zeros(1, self.hidden_dim, x.size(2), x.size(3))
# Concatenate inputs along channel dimension
combined_input = torch.cat([x, hidden_state], dim=1)
# Compute gates
gate_activations = self.conv_gates(combined_input)
reset_gate, update_gate = gate_activations.chunk(2, dim=1)
reset_gate = torch.sigmoid(reset_gate)
update_gate = torch.sigmoid(update_gate)
# Compute candidate hidden state
combined_input_reset = torch.cat([x, hidden_state * reset_gate], dim=1)
candidate = self.conv_candidate(combined_input_reset)
candidate = torch.tanh(candidate)
# Compute new hidden state
new_hidden_state = hidden_state * (1 - update_gate) + candidate * update_gate
return new_hidden_state
```
此代码实现了一个由多个卷积GRU层组成的ConvGRU网络,可以处理输入图像序列。
注意:这里的代码仅供参考,实现细节和输入数据格式等取决于具体的应用场景。
相关推荐
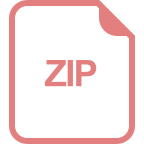
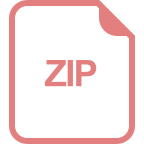














