cnn结合gru的pytorch代码
时间: 2023-05-31 17:19:30 浏览: 410
### 回答1:
以下是一个简单的CNN结合GRU的PyTorch代码示例:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
class CNN_GRU(nn.Module):
def __init__(self, vocab_size, embedding_dim, hidden_dim, output_dim, num_filters, filter_sizes, dropout):
super().__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.convs = nn.ModuleList([
nn.Conv2d(in_channels=1, out_channels=num_filters, kernel_size=(fs, embedding_dim))
for fs in filter_sizes
])
self.gru = nn.GRU(num_filters * len(filter_sizes), hidden_dim, bidirectional=True)
self.fc = nn.Linear(hidden_dim * 2, output_dim)
self.dropout = nn.Dropout(dropout)
def forward(self, text):
# text = [sent len, batch size]
embedded = self.embedding(text)
# embedded = [sent len, batch size, emb dim]
embedded = embedded.unsqueeze(1)
# embedded = [batch size, 1, sent len, emb dim]
conved = [F.relu(conv(embedded)).squeeze(3) for conv in self.convs]
# conved_n = [batch size, num_filters, sent len - filter_sizes[n] + 1]
pooled = [F.max_pool1d(conv, conv.shape[2]).squeeze(2) for conv in conved]
# pooled_n = [batch size, num_filters]
cat = self.dropout(torch.cat(pooled, dim=1))
# cat = [batch size, num_filters * len(filter_sizes)]
_, hidden = self.gru(cat.unsqueeze())
# hidden = [2, batch size, hidden dim]
hidden = self.dropout(torch.cat((hidden[-2,:,:], hidden[-1,:,:]), dim=1))
# hidden = [batch size, hidden dim * 2]
return self.fc(hidden)
```
这个模型包含一个嵌入层,一个卷积层,一个GRU层和一个全连接层。输入的文本首先通过嵌入层进行嵌入,然后通过卷积层进行卷积,再通过池化层进行池化,最后通过GRU层进行序列建模。最后一个隐藏状态被输入到全连接层中,以生成最终的输出。
### 回答2:
在PyTorch中,结合CNN和GRU的代码可以用于实现图像分类任务。这种结合可以有效地处理时序数据和图像数据,用于识别视频帧和动态手势等任务。下面是一个简单的CNN-GRU模型的示例代码。
首先,需要在PyTorch中导入必要的库和模块。
```python
import torch
import torch.nn as nn
import torch.optim as optim
import torch.nn.functional as F
from torch.autograd import Variable
```
接下来,定义模型的结构。这个模型使用两个卷积层和两个池化层来处理图像数据,并使用一个GRU层来处理序列数据。最后,通过一个全连接层将两部分数据进行融合,输出分类结果。
```python
class CNN_GRU_Model(nn.Module):
def __init__(self):
super(CNN_GRU_Model, self).__init__()
# CNN Layers
self.conv1 = nn.Conv2d(3, 32, kernel_size=3)
self.pool1 = nn.MaxPool2d(kernel_size=2)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3)
self.pool2 = nn.MaxPool2d(kernel_size=2)
# GRU Layer
self.gru = nn.GRU(input_size=64, hidden_size=128, batch_first=True)
# Fully Connected Layers
self.fc1 = nn.Linear(128, 64)
self.fc2 = nn.Linear(64, 10)
def forward(self, x):
# Convert 3-channel image to 64-channel feature maps
x = F.relu(self.conv1(x))
x = self.pool1(x)
x = F.relu(self.conv2(x))
x = self.pool2(x)
# Flatten 64-channel feature maps to a 1D vector for GRU input
x = x.view(x.size(0), x.size(1), -1)
x = x.permute(0, 2, 1)
# GRU layer for sequence modeling
x, _ = self.gru(x)
# Fully Connected Layers for Classification
x = F.relu(self.fc1(x[:, -1, :]))
x = self.fc2(x)
return x
```
然后,定义训练数据、测试数据、优化器和损失函数。
```python
# Load CIFAR10 Dataset
train_dataset = torchvision.datasets.CIFAR10(root='./data', train=True, download=True, transform=transforms.ToTensor())
test_dataset = torchvision.datasets.CIFAR10(root='./data', train=False, download=True, transform=transforms.ToTensor())
# Create Data Loaders
train_loader = DataLoader(train_dataset, batch_size=64, shuffle=True)
test_loader = DataLoader(test_dataset, batch_size=64, shuffle=False)
# Define Optimizer and Loss Function
model = CNN_GRU_Model()
optimizer = optim.Adam(model.parameters(), lr=0.001)
criterion = nn.CrossEntropyLoss()
```
最后,进行模型的训练和测试。
```python
# Training Loop
for epoch in range(5):
for i, (inputs, labels) in enumerate(train_loader):
# Forward + Backward + Optimize
optimizer.zero_grad()
outputs = model(inputs)
loss = criterion(outputs, labels)
loss.backward()
optimizer.step()
if (i+1) % 100 == 0:
print ('Epoch [%d/%d], Iter [%d/%d] Loss: %.4f'
%(epoch+1, 5, i+1, len(train_dataset)//64, loss.item()))
# Test the Model
correct = 0
total = 0
for inputs, labels in test_loader:
outputs = model(inputs)
_, predicted = torch.max(outputs.data, 1)
total += labels.size(0)
correct += (predicted == labels).sum()
print('Test Accuracy of the model on the 10000 test images: %.2f %%' % (100.0 * correct / total))
```
总体来说,这个代码演示了如何利用PyTorch结合CNN和GRU的技术来进行图像分类任务。通过对序列和图像两部分数据的处理,可以实现更加复杂的识别任务。
### 回答3:
代码如下:
```python
import torch
import torch.nn as nn
class CNN_GRU(nn.Module):
def __init__(self, embedding_dim, hidden_dim, vocab_size, num_classes, kernel_sizes, num_filters, dropout_prob):
super(CNN_GRU, self).__init__()
self.embedding = nn.Embedding(vocab_size, embedding_dim)
self.convs = nn.ModuleList([nn.Conv2d(1, num_filters, (k, embedding_dim)) for k in kernel_sizes])
self.gru = nn.GRU(num_filters * len(kernel_sizes), hidden_dim, bidirectional=True)
self.fc = nn.Linear(hidden_dim * 2, num_classes)
self.dropout = nn.Dropout(dropout_prob)
def forward(self, x):
x = self.embedding(x)
x = x.unsqueeze(1) # batch_size, 1, seq_len, emb_dim
x = [torch.relu(conv(x)).squeeze(3) for conv in self.convs]
x = [nn.functional.max_pool1d(conv, conv.shape[2]).squeeze(2) for conv in x]
x = torch.cat(x, dim=1) # batch_size, num_filters * len(kernel_sizes)
x = x.unsqueeze(0) # 1, batch_size, num_filters * len(kernel_sizes)
x, hidden = self.gru(x)
x = self.dropout(x)
x = x.squeeze(0) # batch_size, hidden_size * 2
x = self.fc(x)
return x
```
以上代码定义了一个名为 CNN_GRU 的类,该类继承自 nn.Module。
初始化方法中包含了模型的参数:
- embedding_dim:词向量维度
- hidden_dim:GRU 的隐藏层维度
- vocab_size:词汇表大小
- num_classes:分类数目
- kernel_sizes:卷积核大小列表
- num_filters:卷积核数量
- dropout_prob:dropout 概率
在初始化方法中,定义了词嵌入层、一维卷积层、GRU 层、全连接层以及 dropout 层。
在前向方法中,首先对输入 x 进行词嵌入,然后将词嵌入的输出增加一维,即 batch_size, 1, seq_len, emb_dim。
接着使用一维卷积层对词嵌入的输出进行卷积,得到卷积层的输出,再对该输出进行最大池化,得到每个卷积核对应的特征图的最大值,最后将所有卷积核的最大值拼接起来(在 dim=1 的维度上),得到一个特征向量。
接下来,将特征向量增加一维 batch_size,并输入到 GRU 层中。GRU 层的输出和隐藏状态将作为下一个时刻的输入,最后仅取最后一层的输出,通过全连接层得到分类结果。
最后,添加了 dropout 层来缓解过拟合问题。
相关推荐
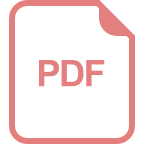
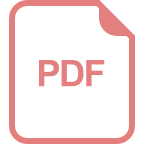
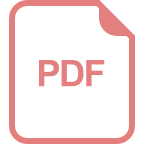














