C++继承和派生练习(一)--关于从people(人员)类派生出student(学生)类等
时间: 2023-08-28 20:03:44 浏览: 80
好的,你的问题是关于从people(人员)类派生出student(学生)类等。我很乐意为你解答这个问题。
在面向对象的编程中,继承是一种非常重要的概念。利用继承,我们可以从一个类派生出一个新的类,并且新类可以继承原类的所有属性和方法。在这里,我们可以用一个人员类来作为基类,然后从这个类派生出学生类等。
下面是一个简单的示例代码,展示了如何从人员类派生出学生类:
```c++
#include <iostream>
#include <string>
using namespace std;
// 定义人员类
class People {
public:
string name;
int age;
string gender;
void showInfo() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
}
};
// 定义学生类,从人员类派生而来
class Student : public People {
public:
string school;
string major;
void showInfo() {
cout << "Name: " << name << endl;
cout << "Age: " << age << endl;
cout << "Gender: " << gender << endl;
cout << "School: " << school << endl;
cout << "Major: " << major << endl;
}
};
int main() {
// 创建一个人员对象
People p;
p.name = "Tom";
p.age = 20;
p.gender = "Male";
p.showInfo();
// 创建一个学生对象
Student s;
s.name = "Lucy";
s.age = 18;
s.gender = "Female";
s.school = "Tsinghua University";
s.major = "Computer Science";
s.showInfo();
return 0;
}
```
在这个示例代码中,我们定义了一个人员类 People,它包含了三个属性:姓名、年龄和性别,并且有一个展示信息的方法 showInfo。接着,我们从 People 类派生出了一个学生类 Student,它包含了两个额外的属性:学校和专业,并且同样有一个展示信息的方法 showInfo。在 main 函数中,我们分别创建了一个人员对象和一个学生对象,并且分别调用了它们的 showInfo 方法。
需要注意的是,我们在定义学生类时使用了 public 关键字来继承 People 类。这意味着从 People 类继承而来的属性和方法在 Student 类中都是 public 的。如果我们使用 private 或 protected 关键字来继承 People 类,那么从 People 类继承而来的属性和方法将会变成相应的访问级别。
希望这个示例代码能够帮助你更好地理解从人员类派生出学生类的概念。
相关推荐
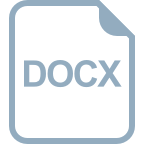
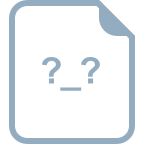
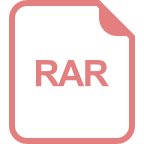








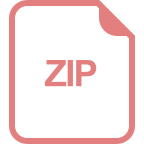
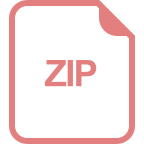
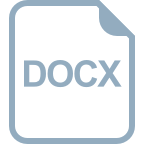
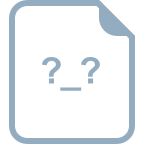
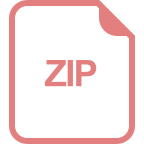
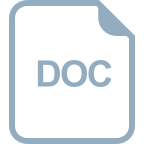
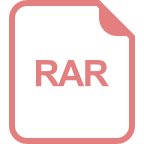
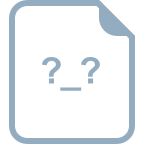