基于pytorch,写LSTM深度学习基于时间序列A预测序列B的代码
时间: 2023-03-01 17:00:08 浏览: 93
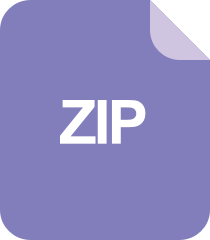
基于pytorch的LSTM多变量多输出时间序列预测使用例

Here is a simple example code for using an LSTM in PyTorch to predict sequence B based on sequence A:
```
import torch
import torch.nn as nn
class LSTM(nn.Module):
def __init__(self, input_size, hidden_size, num_layers, output_size):
super(LSTM, self).__init__()
self.hidden_size = hidden_size
self.num_layers = num_layers
self.lstm = nn.LSTM(input_size, hidden_size, num_layers, batch_first=True)
self.fc = nn.Linear(hidden_size, output_size)
def forward(self, x):
h0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size)
c0 = torch.zeros(self.num_layers, x.size(0), self.hidden_size)
out, (hn, cn) = self.lstm(x, (h0, c0))
out = self.fc(out[:, -1, :])
return out
input_size = 1
hidden_size = 128
num_layers = 2
output_size = 1
model = LSTM(input_size, hidden_size, num_layers, output_size)
```
In this example, `input_size` represents the number of features in sequence A, `hidden_size` represents the number of hidden units in the LSTM layer, `num_layers` represents the number of LSTM layers, and `output_size` represents the number of outputs in sequence B. The `forward` method takes an input sequence `x` and returns the predicted sequence.
You can then train the model using the input and target sequences of A and B, respectively. Here's a simple example of how you might do this:
```
criterion = nn.MSELoss()
optimizer = torch.optim.Adam(model.parameters(), lr=0.001)
for epoch in range(num_epochs):
outputs = model(inputs)
loss = criterion(outputs, targets)
optimizer.zero_grad()
loss.backward()
optimizer.step()
```
In this example, `num_epochs` is the number of training epochs, `inputs` is a tensor representing the input sequence A, and `targets` is a tensor representing the target sequence B. The `MSELoss` function computes the mean squared error between the predicted and target sequences. The optimizer is used to update the model parameters based on the computed gradients.
阅读全文
相关推荐
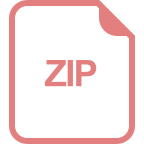
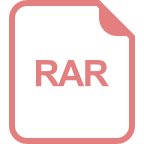














