逻辑回归的特征缩放底层函数实现
时间: 2023-07-06 17:43:53 浏览: 109
逻辑回归的特征缩放底层函数实现包括以下步骤:
1. 计算每个特征的均值和标准差。
2. 对每个特征进行标准化,即将每个特征的值减去对应的均值,然后除以对应的标准差。
3. 将标准化后的特征作为输入进行逻辑回归模型的训练。
具体实现可以参考以下代码:
```python
def feature_scaling(X):
mu = np.mean(X, axis=0)
sigma = np.std(X, axis=0)
X_norm = (X - mu) / sigma
return X_norm, mu, sigma
def sigmoid(z):
return 1 / (1 + np.exp(-z))
def cost_function(theta, X, y):
m = len(y)
h = sigmoid(X.dot(theta))
J = -1/m * (y.T.dot(np.log(h)) + (1-y).T.dot(np.log(1-h)))
grad = 1/m * X.T.dot(h-y)
return J, grad
def gradient_descent(theta, X, y, alpha, num_iters):
m = len(y)
J_history = []
for i in range(num_iters):
J, grad = cost_function(theta, X, y)
theta = theta - alpha * grad
J_history.append(J)
return theta, J_history
# 使用样例数据进行测试
X = np.array([[1, 2104, 5],
[1, 1416, 3],
[1, 1534, 3],
[1, 852, 2]])
y = np.array([[1], [0], [0], [1]])
X_norm, mu, sigma = feature_scaling(X)
theta = np.zeros((X_norm.shape[1], 1))
alpha = 0.01
num_iters = 1000
theta, J_history = gradient_descent(theta, X_norm, y, alpha, num_iters)
```
其中,`feature_scaling` 函数用于对特征进行标准化,`sigmoid` 函数用于计算逻辑回归的预测值,`cost_function` 函数用于计算代价函数和梯度,`gradient_descent` 函数用于执行梯度下降算法进行模型训练。
阅读全文
相关推荐
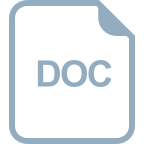
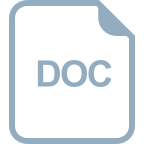
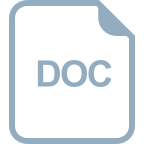
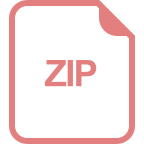
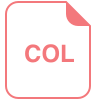
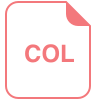
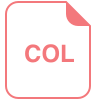
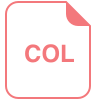
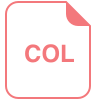
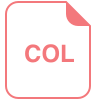
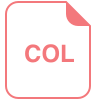
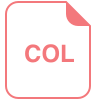
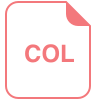
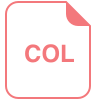
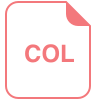
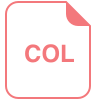
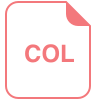
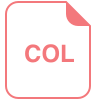