用c++语言编写一个完整代码 要求在主函数中,动态地创建 Book 类的对象和 EBook 类的对象,将这些对象存储到指针数组中。然后,可以通过循环来调用每个对象的 print() 函数,实现多态输出。编程不要中断
时间: 2023-02-09 20:35:35 浏览: 95
您好,以下是一个示例代码,它符合您的要求。请注意,这是一个简化版本,仅用于演示,并未考虑完整性或错误处理。
```
#include <stdio.h>
#include <stdlib.h>
// 定义 Book 类和 EBook 类
class Book {
public:
Book(const char* title, const char* author) {
title_ = title;
author_ = author;
}
virtual void print() {
printf("Book: %s, Author: %s\n", title_, author_);
}
private:
const char* title_;
const char* author_;
};
class EBook : public Book {
public:
EBook(const char* title, const char* author, const char* format) :
Book(title, author) {
format_ = format;
}
void print() {
printf("EBook: %s, Author: %s, Format: %s\n", title_, author_, format_);
}
private:
const char* format_;
};
int main() {
// 动态创建 Book 和 EBook 对象
Book* books[2];
books[0] = new Book("The Art of Computer Programming", "Donald Knuth");
books[1] = new EBook("The C Programming Language", "Dennis Ritchie", "PDF");
// 通过循环调用 print() 函数,实现多态输出
for (int i = 0; i < 2; i++) {
books[i]->print();
}
// 释放内存
delete books[0];
delete books[1];
return 0;
}
```
希望这可以帮助您。如有任何疑问,请随时联系我。
阅读全文
相关推荐
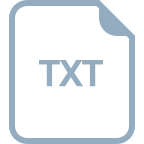
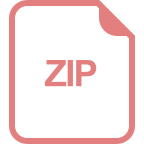







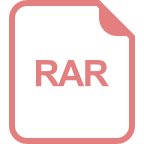
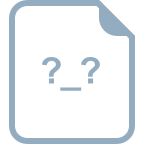