请实现链栈的基本操作,包括初始化、判断栈空、栈满,求栈长,清空栈,销毁栈,进栈,出栈,取栈顶元素。
时间: 2024-04-30 14:14:04 浏览: 266
以下是链栈的基本操作实现:
1.初始化
```c
LinkedStack Init_LinkedStack() {
LinkedStack top = (LinkedStack)malloc(sizeof(LinkedStackNode)); top->next = NULL;
return top;
}
```
2.判断栈空
```c
int IsEmpty_LinkedStack(LinkedStack top) {
return top->next == NULL;
}
```
3.判断栈满
链栈不需要判断栈满。
4.求栈长
```c
int Length_LinkedStack(LinkedStack top) {
int len = 0;
LinkedStackNode *p = top->next;
while (p) {
len++;
p = p->next;
}
return len;
}
```
5.清空栈
```c
void Clear_LinkedStack(LinkedStack top) {
LinkedStackNode *p = top->next;
while (p) {
top->next = p->next;
free(p);
p = top->next;
}
}
```
6.销毁栈
```c
void Destroy_LinkedStack(LinkedStack top) {
Clear_LinkedStack(top);
free(top);}
```
7.进栈
```c
void Push_LinkedStack(LinkedStack top, elemtype x) {
LinkedStackNode *node = (LinkedStackNode *)malloc(sizeof(LinkedStackNode));
node->data = x;
node->next = top->next;
top->next = node;
}
```
8.出栈
```c
int Pop_LinkedStack(LinkedStack top, elemtype *x) {
LinkedStackNode *node;
if (top->next == NULL) {
return 0;
} else {
node = top->next;
*x = node->data;
top->next = node->next;
free(node);
return 1;
}
}
```
9.取栈顶元素
```c
int GetTop_LinkedStack(LinkedStack top) {
if (top->next) {
return top->next->data;
}
return -1;
}
```
阅读全文
相关推荐



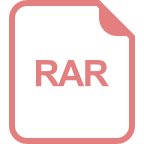

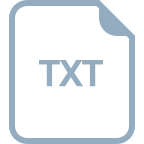
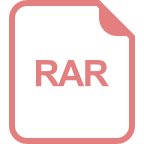
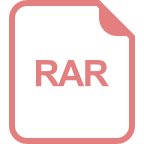
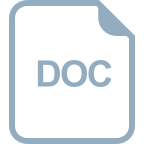
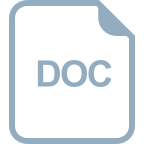
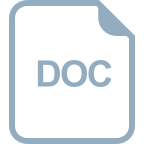
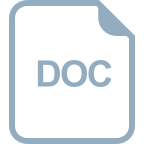
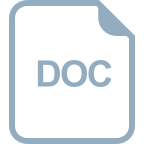
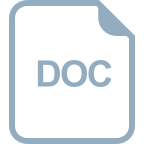
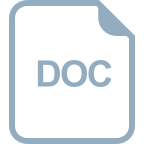
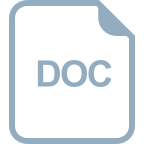