vue使用$refs设置div高度
时间: 2023-06-27 21:03:44 浏览: 236
可以使用 `$refs` 来获取到DOM元素并设置它的高度,例如:
```html
<template>
<div ref="myDiv"></div>
</template>
<script>
export default {
mounted() {
this.$refs.myDiv.style.height = "200px";
}
}
</script>
```
在 `mounted()` 钩子函数中,我们通过 `this.$refs` 来获取到 `myDiv` 元素,并通过 `style` 属性来设置它的高度为 `200px`。当然,你可以根据需要设置任何高度值。
相关问题
组合式vue使用$refs
在Vue 3中,由于使用了组合式API,所以无法像Vue 2中那样使用this.$refs来访问组件的属性或方法。取而代之的是使用getCurrentInstance方法来获取当前实例,并通过该实例的$refs属性来访问组件的ref属性。例如,在template标签中使用ref属性绑定一个组件:
```
<template>
<div>
<div>
<van-swipe class="my-swipe" ref="swipe" width="100%">
<van-swipe-item @click="toExhibitionPages">
<div>
<img src="./imgs/1.jpg" />
</div>
</van-swipe-item>
</van-swipe>
</div>
<div @click="nextImg">
<van-icon name="arrow" />
</div>
<div @click="preImg">
<van-icon name="arrow-left" />
</div>
</div>
</template>
<script setup>
import { ref, getCurrentInstance } from "vue";
const { proxy } = getCurrentInstance();
function nextImg() {
proxy.$refs.swipe.next();
}
function preImg() {
proxy.$refs.swipe.prev();
}
</script>
```
在这个例子中,我们通过getCurrentInstance方法获取到当前实例的proxy属性,并使用$refs属性来访问ref属性为"swipe"的组件。例如,我们可以通过proxy.$refs.swipe调用该组件的next和prev方法来切换图片。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [在vue3组合式api中使用vue2的$refs](https://blog.csdn.net/weixin_55869175/article/details/124827021)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [vue3使用$refs](https://blog.csdn.net/weixin_52164116/article/details/129683079)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [计算机程序设计语言课程设计(VUE.js)及实践项目的例子.txt](https://download.csdn.net/download/weixin_44609920/88236928)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
vue使用$refs移除监听事件
根据提供的引用内容,没有提到vue使用$refs移除监听事件的方法。但是可以使用vm.$off()方法来移除自定义事件监听器,具体使用方法如下:
1. 在需要移除监听器的组件中,使用ref属性给组件命名。
2. 在组件中使用$on()方法监听自定义事件。
3. 在需要移除监听器的时候,使用$refs获取组件实例,然后使用$off()方法移除监听器。
例如,假设我们有一个名为"my-component"的组件,我们可以在父组件中使用以下代码来移除监听器:
```
<template>
<div>
<my-component ref="myComponent"></my-component>
<button @click="removeListener">移除监听器</button>
</div>
</template>
<script>
export default {
methods: {
removeListener() {
this.$refs.myComponent.$off('customEvent', this.handleCustomEvent)
},
handleCustomEvent() {
// 处理自定义事件
}
}
}
</script>
```
在上面的代码中,我们在父组件中使用了"my-component"组件,并给它设置了一个ref属性。然后,在父组件的方法中,我们使用$refs获取了"my-component"组件的实例,并使用$off()方法移除了它的自定义事件监听器。
阅读全文
相关推荐
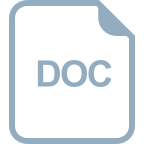
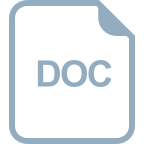
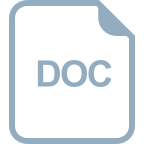
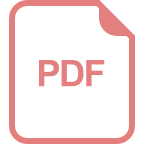
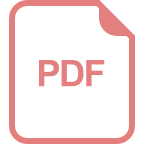
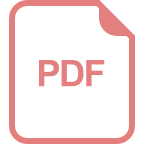
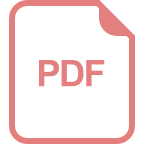









