推箱子代码c语言
时间: 2023-08-12 14:21:18 浏览: 169
下面是一个简单的推箱子游戏的C语言代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <conio.h>
#define ROW 10
#define COL 10
#define WALL '#'
#define BOX '$'
#define GOAL '.'
#define MAN '@'
char level[ROW][COL+1] = {
"##########",
"# #",
"# $ #",
"# . #",
"# # #",
"# ##### #",
"# @ #",
"# #",
"# #",
"##########",
};
int manRow, manCol; // 人所在的行和列
void drawLevel() { // 画出当前关卡的地图
system("cls"); // 清屏
for (int i = 0; i < ROW; i++) {
printf("%s\n", level[i]);
}
}
void findMan() { // 找出人所在的位置
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (level[i][j] == MAN) {
manRow = i;
manCol = j;
return;
}
}
}
}
void moveBox(int row1, int col1, int row2, int col2) { // 移动箱子
level[row2][col2] = BOX;
level[row1][col1] = (level[row1][col1] == GOAL) ? GOAL : ' ';
}
int pushBox(int row, int col, int dirRow, int dirCol) { // 推箱子
if (level[row+dirRow][col+dirCol] == WALL || level[row+dirRow][col+dirCol] == BOX) {
return 0;
}
if (level[row+dirRow][col+dirCol] == GOAL) {
moveBox(row, col, row+dirRow, col+dirCol);
return 1;
}
moveBox(row, col, row+dirRow, col+dirCol);
return 1;
}
void moveMan(int dirRow, int dirCol) { // 移动人
if (level[manRow+dirRow][manCol+dirCol] == WALL) {
return;
}
if (level[manRow+dirRow][manCol+dirCol] == BOX) {
if (pushBox(manRow+dirRow, manCol+dirCol, dirRow, dirCol)) {
manRow += dirRow;
manCol += dirCol;
}
} else {
manRow += dirRow;
manCol += dirCol;
}
}
int checkWin() { // 检查是否胜利
for (int i = 0; i < ROW; i++) {
for (int j = 0; j < COL; j++) {
if (level[i][j] == GOAL || level[i][j] == BOX) {
return 0;
}
}
}
return 1;
}
int main() {
char key;
drawLevel();
findMan();
while (1) {
key = getch();
switch (key) {
case 'w': moveMan(-1, 0); break; // 上
case 's': moveMan(1, 0); break; // 下
case 'a': moveMan(0, -1); break; // 左
case 'd': moveMan(0, 1); break; // 右
case 'q': exit(0); // 退出游戏
default: break;
}
drawLevel();
if (checkWin()) {
printf("You win!\n");
break;
}
}
return 0;
}
```
这个游戏地图使用一个二维字符数组 `level` 来表示,每个元素表示一个格子的类型,其中 `#` 表示墙,`$` 表示箱子,`.` 表示目标点,`@` 表示人。`manRow` 和 `manCol` 分别表示人所在的行和列。游戏的流程是不断等待玩家输入方向键,然后根据方向键的方向移动人或者推动箱子。当所有的箱子都被推到目标点上时,游戏胜利。
阅读全文
相关推荐

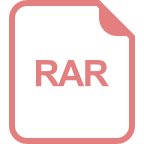
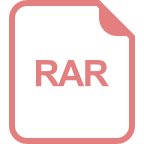












