写个推箱子的c语言代码
时间: 2023-09-21 16:06:33 浏览: 81
### 回答1:
下面是一份简单的推箱子代码:
```
#include <stdio.h>
#include <stdlib.h>
#define ROW 5
#define COL 5
char map[ROW][COL]={
{'#','#','#','#','#'},
{'#','S','.','.','#'},
{'#','.','#','.','#'},
{'#','.','.','T','#'},
{'#','#','#','#','#'}
};
void printMap()
{
int i, j;
for(i=0; i<ROW; i++)
{
for(j=0; j<COL; j++)
{
printf("%c ", map[i][j]);
}
printf("\n");
}
printf("\n");
}
void move(int x, int y, int x2, int y2)
{
map[x2][y2]=map[x][y];
map[x][y]='.';
}
int main()
{
int x, y, x2, y2;
printMap();
while(1)
{
printf("请输入要移动的方向(W/A/S/D):");
char c=getchar();
getchar();
switch(c)
{
case 'w':
case 'W':
x=0; y=0;
for(x=0; x<ROW; x++)
{
for(y=0; y<COL; y++)
{
if(map[x][y]=='S')
{
if(map[x-1][y]!='#')
{
if(map[x-1][y]=='.')
{
move(x, y, x-1, y);
}
else
{
if(map[x-2][y]=='.')
{
move(x, y, x-2, y);
move(x-1, y, x-1, y);
}
}
}
break;
}
}
if(map[x][y]=='S')
{
break;
}
}
break;
case 's':
case 'S':
x=0; y=0;
for(x=0; x<ROW; x++)
{
for(y=0; y<COL; y++)
{
if(map[x][y]=='S')
{
if(map[x+1][y]!='#')
{
if(map[x+1][y]=='.')
{
move(x, y, x+1, y);
}
else
### 回答2:
推箱子是一种经典的益智游戏,玩家需要将箱子推到指定位置,达成目标。以下是一个简单的推箱子的C语言代码实现:
```c
#include <stdio.h>
#include <conio.h>
#define MAP_SIZE 5 // 地图大小
#define PLAYER 'P' // 玩家表示
#define BOX 'B' // 箱子表示
#define TARGET 'T' // 目标位置表示
#define EMPTY ' ' // 空格表示
// 定义地图
const char map[MAP_SIZE][MAP_SIZE] = {
{EMPTY, EMPTY, EMPTY, TARGET, EMPTY},
{EMPTY, EMPTY, EMPTY, TARGET, EMPTY},
{EMPTY, EMPTY, EMPTY, EMPTY, EMPTY},
{EMPTY, PLAYER, BOX, EMPTY, EMPTY},
{EMPTY, EMPTY, EMPTY, EMPTY, EMPTY}
};
// 定义玩家位置
int playerRow = 3;
int playerCol = 1;
// 检查是否胜利
int checkWin() {
int i, j;
for (i = 0; i < MAP_SIZE; i++) {
for (j = 0; j < MAP_SIZE; j++) {
if (map[i][j] == TARGET || map[i][j] == BOX) {
return 0; // 存在未到达目标位置的箱子或目标位置
}
}
}
return 1; // 所有箱子都到达了目标位置,胜利
}
// 打印地图
void printMap() {
int i, j;
for (i = 0; i < MAP_SIZE; i++) {
for (j = 0; j < MAP_SIZE; j++) {
if (i == playerRow && j == playerCol) {
printf("%c ", PLAYER);
} else {
printf("%c ", map[i][j]);
}
}
printf("\n");
}
}
int main() {
char move;
int newRow, newCol;
while (1) {
system("cls"); // 清屏
printMap();
if (checkWin()) {
printf("恭喜,您胜利了!\n");
break;
}
move = getch();
// 根据按键计算新位置
newRow = playerRow;
newCol = playerCol;
switch (move) {
case 'w':
newRow--;
break;
case 's':
newRow++;
break;
case 'a':
newCol--;
break;
case 'd':
newCol++;
break;
default:
break;
}
// 检查新位置是否合法,不合法则继续循环
if (newRow < 0 || newRow >= MAP_SIZE ||
newCol < 0 || newCol >= MAP_SIZE ||
map[newRow][newCol] == TARGET) {
continue;
}
// 移动箱子
if (map[newRow][newCol] == BOX) {
int boxRow = newRow + (newRow - playerRow);
int boxCol = newCol + (newCol - playerCol);
if (boxRow < 0 || boxRow >= MAP_SIZE ||
boxCol < 0 || boxCol >= MAP_SIZE ||
map[boxRow][boxCol] == BOX || map[boxRow][boxCol] == TARGET) {
continue;
}
map[boxRow][boxCol] = BOX;
}
// 移动玩家
map[playerRow][playerCol] = EMPTY;
playerRow = newRow;
playerCol = newCol;
map[playerRow][playerCol] = PLAYER;
}
return 0;
}
```
以上代码实现了一个5x5的地图,玩家使用WASD键控制上下左右移动,在移动过程中判断并移动箱子,直到所有箱子到达目标位置为止。代码通过字符表示元素,在控制台打印地图,使用`getch()`函数获取键盘输入。游戏结束后通过判断是否胜利来决定显示胜利信息。
### 回答3:
推箱子是一种益智游戏,要求玩家将箱子推到指定位置。下面是一个简单的用C语言编写的推箱子游戏代码:
```c
#include <stdio.h>
#include <stdlib.h>
#define WIDTH 9
#define HEIGHT 7
int main()
{
// 定义游戏地图数组
char map[HEIGHT][WIDTH] = {"#########",
"# # #",
"# X P #",
"# ###",
"#########",
};
int playerX = 4; // 玩家的初始位置
int playerY = 2;
int hasBox = 0; // 玩家是否有箱子
// 游戏主循环
while (1)
{
// 打印游戏地图
system("clear");
for (int i = 0; i < HEIGHT; i++)
{
for (int j = 0; j < WIDTH; j++)
{
printf("%c", map[i][j]);
}
printf("\n");
}
// 判断游戏是否胜利
if (map[2][6] == 'X')
{
printf("You win!\n");
break;
}
// 接受玩家输入移动方向
char direction;
printf("Please input direction: ");
scanf(" %c", &direction);
int nextX = playerX;
int nextY = playerY;
// 计算下一步位置
switch (direction)
{
case 'w': // 上
nextY--;
break;
case 's': // 下
nextY++;
break;
case 'a': // 左
nextX--;
break;
case 'd': // 右
nextX++;
break;
default:
break;
}
// 判断下一步是否可移动
char nextCell = map[nextY][nextX];
if (nextCell == ' ' || nextCell == 'X')
{
// 更新地图和玩家位置
if (hasBox == 1)
{
map[playerY][playerX] = 'X';
map[nextY][nextX] = 'P';
hasBox = 0;
}
else
{
map[playerY][playerX] = ' ';
map[nextY][nextX] = 'P';
}
playerX = nextX;
playerY = nextY;
}
else if (nextCell == '#')
{
// 碰到墙壁,不能移动
printf("Cannot move!\n");
}
else if (nextCell == 'X')
{
// 推动箱子
int afterNextX = nextX;
int afterNextY = nextY;
// 计算箱子下一步位置
switch (direction)
{
case 'w': // 上
afterNextY--;
break;
case 's': // 下
afterNextY++;
break;
case 'a': // 左
afterNextX--;
break;
case 'd': // 右
afterNextX++;
break;
default:
break;
}
char afterNextCell = map[afterNextY][afterNextX];
if (afterNextCell == ' ' || afterNextCell == 'X')
{
// 更新地图和玩家位置
map[playerY][playerX] = ' ';
map[nextY][nextX] = 'P';
map[afterNextY][afterNextX] = 'X';
playerX = nextX;
playerY = nextY;
hasBox = 1;
}
else if (afterNextCell == '#')
{
// 箱子后面是墙壁,不能推动
printf("Cannot move!\n");
}
}
}
return 0;
}
```
游戏地图的数组`map`表示了地图的布局,'P'代表玩家,'X'代表箱子,'#'代表墙壁。
玩家可以通过输入方向键来操作玩家移动,玩家可以推动箱子,但不能穿墙。
请在编译运行后,根据提示输入方向,使玩家推动箱子到指定位置。如果成功推动到指定位置,会输出"You win!"。注意,需要在类Unix系统下编译运行,如Linux或macOS。
相关推荐
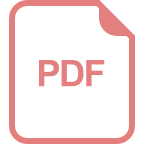














