Qt用QPainter和三个左边点画出圆弧
时间: 2023-11-22 14:54:06 浏览: 279
可以使用QPainter的drawArc()函数来画出圆弧,需要指定圆弧所在的矩形区域和起始角度、跨越角度。以下是一个示例代码,其中三个左边点为(50,50)、(50,100)、(50,150):
```cpp
QPainter painter(this);
QPen pen(Qt::black, 2);
painter.setPen(pen);
QRectF rect(50, 50, 100, 100);
int startAngle = 30 * 16; // 起始角度,单位为1/16度
int spanAngle = 120 * 16; // 跨越角度,单位为1/16度
painter.drawArc(rect, startAngle, spanAngle);
```
这段代码会在当前widget上绘制一个矩形区域为(50,50,100,100),起始角度为30度,跨越角度为120度的圆弧。你可以根据具体需求调整矩形区域和角度参数。
相关问题
Qt用椭圆的5个点画椭圆的外接矩形
在Qt中,可以使用QPainter来绘制椭圆的外接矩形。以下是一个基本的示例代码:
```
QPainter painter(this);
QRectF ellipseRect(50, 50, 100, 80);
painter.drawEllipse(ellipseRect);
QRectF boundingRect = ellipseRect.normalized();
QPointF topLeft = boundingRect.topLeft();
QPointF topRight = boundingRect.topRight();
QPointF bottomLeft = boundingRect.bottomLeft();
QPointF bottomRight = boundingRect.bottomRight();
// 画出矩形的五个点
painter.drawPoint(topLeft);
painter.drawPoint(topRight);
painter.drawPoint(bottomLeft);
painter.drawPoint(bottomRight);
painter.drawPoint(boundingRect.center());
// 画出矩形
painter.drawRect(boundingRect);
```
在这个示例中,我们首先绘制了一个椭圆,然后通过调用`normalized()`方法获取其外接矩形,并且计算出了矩形的五个点。最后,我们使用`drawRect()`方法绘制了矩形,并使用`drawPoint()`方法绘制了矩形的五个点。
qt用QPainter显示图片
在Qt框架中,`QPainter`类是一个强大的绘图工具,它可以用于绘制各种图形元素,包括文本、线条、形状和图像。要使用`QPainter`显示图片,通常你会在`QWidget`的子类中重写`paintEvent`函数,并在此函数中使用`QPainter`对象来进行绘制。
以下是一个简单的示例,展示了如何在一个自定义的`QWidget`子类中使用`QPainter`来显示一张图片:
```cpp
#include <QPainter>
#include <QPixmap>
class ImageWidget : public QWidget {
protected:
void paintEvent(QPaintEvent *event) override {
QPainter painter(this);
// 加载图片
QPixmap pixmap(":/path/to/image.png");
// 检查图片是否加载成功
if (!pixmap.isNull()) {
// 在窗口的中心绘制图片
int x = (width() - pixmap.width()) / 2;
int y = (height() - pixmap.height()) / 2;
painter.drawPixmap(x, y, pixmap);
}
}
};
```
在这个示例中,我们首先创建了一个继承自`QWidget`的`ImageWidget`类。在这个类中,我们重写了`paintEvent`函数。在`paintEvent`函数内部,我们创建了一个`QPainter`对象用于绘图,并使用`QPixmap`类来加载图片。使用`drawPixmap`函数,我们将图片绘制到窗口的中心位置。
需要注意的是,图片路径`":/path/to/image.png"`通常是一个资源路径,你可以通过Qt的资源系统来管理你的图片文件。这种方式有助于简化资源的管理,并且可以将资源文件打包到应用程序中。
阅读全文
相关推荐




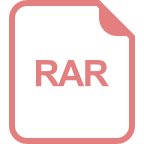








