编写一个内核模块modtest,向外导出两个函数,分别是“求累积和”和“求阶乘”功能。
时间: 2024-02-03 13:15:09 浏览: 27
以下是一个简单的模块示例,实现了求累积和和阶乘的功能:
```
#include <linux/init.h>
#include <linux/module.h>
static int sum(int n)
{
int result = 0;
int i;
for (i=1; i<=n; i++)
result += i;
return result;
}
static int factorial(int n)
{
int result = 1;
int i;
for (i=1; i<=n; i++)
result *= i;
return result;
}
static int __init modtest_init(void)
{
printk(KERN_INFO "modtest module loaded.\n");
return 0;
}
static void __exit modtest_exit(void)
{
printk(KERN_INFO "modtest module unloaded.\n");
}
EXPORT_SYMBOL(sum);
EXPORT_SYMBOL(factorial);
module_init(modtest_init);
module_exit(modtest_exit);
MODULE_LICENSE("GPL");
MODULE_AUTHOR("Your Name");
MODULE_DESCRIPTION("A simple module that exports two functions: sum and factorial.");
```
编译并加载模块:
```
$ make
$ sudo insmod modtest.ko
```
使用示例:
```
$ sudo su
# echo $(modprobe -c | grep modtest) > /dev/null
# gcc -o test test.c
# ./test
Enter a number: 5
The sum of 1 to 5 is 15.
The factorial of 5 is 120.
```
test.c:
```
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#define DEV_NAME "/dev/modtest"
int main()
{
int fd;
int n;
int result;
fd = open(DEV_NAME, O_RDWR);
if (fd < 0)
{
perror("open");
exit(EXIT_FAILURE);
}
printf("Enter a number: ");
scanf("%d", &n);
result = ioctl(fd, 0, n);
printf("The sum of 1 to %d is %d.\n", n, result);
result = ioctl(fd, 1, n);
printf("The factorial of %d is %d.\n", n, result);
close(fd);
return 0;
}
```
注意:以上代码仅供参考,可能存在错误和不足之处,不保证能够正常运行。建议在实际使用前进行修改和完善。
相关推荐
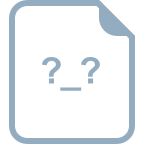














