1. Write a short program that asks for your height in integer inches and then converts your height to feet and inches. Have the program use the underscore character to indicate where to type the response. Also use a const symbolic constant to repre¬sent the conversion factor.
时间: 2023-01-11 18:55:36 浏览: 99
输入身高:
```
const int CONVERSION_FACTOR = 12;
int inches, feet;
cout << "请输入身高(英寸): _";
cin >> inches;
feet = inches / CONVERSION_FACTOR;
inches = inches % CONVERSION_FACTOR;
cout << "你的身高是: " << feet << "英尺" << inches << "英寸" << endl;
```
这段代码提示用户输入身高, 然后使用常量 `CONVERSION_FACTOR` (12) 将身高从英寸转换为英尺和英寸. 使用整数除法来计算英尺, 使用整数取模来计算英寸. 最后, 输出转换后的身高.
相关问题
use c++ 2. Write a program that asks the user for three words and prints out the words in alphabetical order, then the words concatenated in alphabetical order (stored in a fourth string).
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string word1, word2, word3;
string sorted_words, concat_words;
// ask user for three words
cout << "Enter three words: ";
cin >> word1 >> word2 >> word3;
// sort the words in alphabetical order
if (word1 <= word2 && word1 <= word3) {
sorted_words = word1;
if (word2 <= word3) {
sorted_words += " " + word2 + " " + word3;
} else {
sorted_words += " " + word3 + " " + word2;
}
} else if (word2 <= word1 && word2 <= word3) {
sorted_words = word2;
if (word1 <= word3) {
sorted_words += " " + word1 + " " + word3;
} else {
sorted_words += " " + word3 + " " + word1;
}
} else {
sorted_words = word3;
if (word1 <= word2) {
sorted_words += " " + word1 + " " + word2;
} else {
sorted_words += " " + word2 + " " + word1;
}
}
// concatenate the words in alphabetical order
if (word1 <= word2 && word1 <= word3) {
concat_words = word1;
if (word2 <= word3) {
concat_words += word2 + word3;
} else {
concat_words += word3 + word2;
}
} else if (word2 <= word1 && word2 <= word3) {
concat_words = word2;
if (word1 <= word3) {
concat_words += word1 + word3;
} else {
concat_words += word3 + word1;
}
} else {
concat_words = word3;
if (word1 <= word2) {
concat_words += word1 + word2;
} else {
concat_words += word2 + word1;
}
}
// output the sorted and concatenated words
cout << "Sorted words: " << sorted_words << endl;
cout << "Concatenated words: " << concat_words << endl;
return 0;
}
```
Sample output:
```
Enter three words: apple dog cat
Sorted words: apple cat dog
Concatenated words: applecatdog
```
write a c program that receives two integer parameters x and y , prints the value of x times y, then returns x.
Certainly! Here's a C program that receives two integer parameters `x` and `y`, prints the value of `x` times `y`, and then returns `x`:
```c
#include <stdio.h>
int multiplyAndReturnX(int x, int y) {
int result = x * y;
printf("The value of x times y is: %d\n", result);
return x;
}
int main() {
int x, y;
printf("Enter the value of x: ");
scanf("%d", &x);
printf("Enter the value of y: ");
scanf("%d", &y);
int returnValue = multiplyAndReturnX(x, y);
printf("The value of x is: %d\n", returnValue);
return 0;
}
```
In this program, the `multiplyAndReturnX` function takes two parameters `x` and `y`. It calculates the product of `x` and `y` and stores it in the `result` variable. Then, it prints the value of `x` times `y` using `printf`, and finally returns the value of `x`.
In the `main` function, it asks the user to enter the values of `x` and `y`. It calls the `multiplyAndReturnX` function with these values and stores the returned value in `returnValue`. Finally, it prints the value of `x` using `printf`.
相关推荐
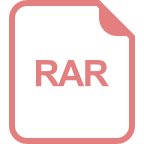
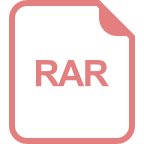
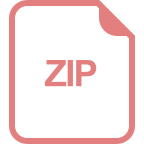













