Write a program that asks the user to enter four numbers, calculate the sum of the front two numbers, the last two numbers, respectively. Finally, prints the sum, product, difference, and quotient of these two sums.
时间: 2024-03-13 19:46:59 浏览: 83
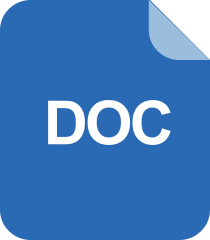
编写一个用输入和输出流运算符进行的输入和输出的程序。从键盘输入两个数,分别对两个数进行加,减,乘和除的运算。
好的,以下是一个符合您要求的 C 语言程序:
```c
#include <stdio.h>
int main() {
// 输入四个数
float a, b, c, d;
printf("Please enter four numbers: ");
scanf("%f %f %f %f", &a, &b, &c, &d);
// 计算前两个数的和
float sum1 = a + b;
// 计算后两个数的和
float sum2 = c + d;
// 输出两个和的结果
printf("Sum of the first two numbers: %.2f\n", sum1);
printf("Sum of the last two numbers: %.2f\n", sum2);
// 计算两个和的乘积、差和商
float product = sum1 * sum2;
float difference = sum1 - sum2;
float quotient = sum1 / sum2;
// 输出结果
printf("Product of two sums: %.2f\n", product);
printf("Difference of two sums: %.2f\n", difference);
printf("Quotient of two sums: %.2f\n", quotient);
return 0;
}
```
程序首先提示用户输入四个数,然后计算前两个数的和和后两个数的和。接着计算两个和的乘积、差和商,并输出结果。程序中使用了 `float` 类型来存储输入的数和计算结果,并使用了 `scanf` 函数来读取用户的输入。
阅读全文
相关推荐
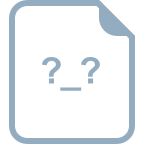
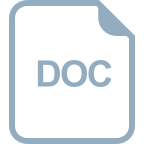
















