use c++ 2. Write a program that asks the user for three words and prints out the words in alphabetical order, then the words concatenated in alphabetical order (stored in a fourth string).
时间: 2023-06-14 09:08:14 浏览: 123
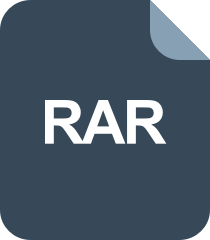
打印2进制的C++程序
```c++
#include <iostream>
#include <string>
using namespace std;
int main() {
string word1, word2, word3;
string sorted_words, concat_words;
// ask user for three words
cout << "Enter three words: ";
cin >> word1 >> word2 >> word3;
// sort the words in alphabetical order
if (word1 <= word2 && word1 <= word3) {
sorted_words = word1;
if (word2 <= word3) {
sorted_words += " " + word2 + " " + word3;
} else {
sorted_words += " " + word3 + " " + word2;
}
} else if (word2 <= word1 && word2 <= word3) {
sorted_words = word2;
if (word1 <= word3) {
sorted_words += " " + word1 + " " + word3;
} else {
sorted_words += " " + word3 + " " + word1;
}
} else {
sorted_words = word3;
if (word1 <= word2) {
sorted_words += " " + word1 + " " + word2;
} else {
sorted_words += " " + word2 + " " + word1;
}
}
// concatenate the words in alphabetical order
if (word1 <= word2 && word1 <= word3) {
concat_words = word1;
if (word2 <= word3) {
concat_words += word2 + word3;
} else {
concat_words += word3 + word2;
}
} else if (word2 <= word1 && word2 <= word3) {
concat_words = word2;
if (word1 <= word3) {
concat_words += word1 + word3;
} else {
concat_words += word3 + word1;
}
} else {
concat_words = word3;
if (word1 <= word2) {
concat_words += word1 + word2;
} else {
concat_words += word2 + word1;
}
}
// output the sorted and concatenated words
cout << "Sorted words: " << sorted_words << endl;
cout << "Concatenated words: " << concat_words << endl;
return 0;
}
```
Sample output:
```
Enter three words: apple dog cat
Sorted words: apple cat dog
Concatenated words: applecatdog
```
阅读全文
相关推荐
















