3. Develop an application that accepts a word from the user and calculates and outputs the number of letters in the word. The application should check that only one word was entered. If more than one word was entered, the application should output a message to the user and repeat to allow them to enter the word again. The application should make use of instantiable classes. Name the instantiable class Word.java.
时间: 2023-11-27 13:50:32 浏览: 50
Here is the code for the Word.java instantiable class:
```java
public class Word {
private String word;
public void setWord(String word) {
this.word = word;
}
public int countLetters() {
return word.length();
}
public boolean isSingleWord() {
return !word.contains(" ");
}
}
```
And here is the code for the main application:
```java
import java.util.Scanner;
public class Main {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
Word word = new Word();
while (true) {
System.out.print("Enter a word: ");
String input = scanner.nextLine();
word.setWord(input);
if (word.isSingleWord()) {
int count = word.countLetters();
System.out.printf("The word \"%s\" has %d letters.%n", input, count);
break;
} else {
System.out.println("Please enter only one word.");
}
}
}
}
```
The main method uses a while loop to continuously prompt the user to enter a word until a single word is entered. The word is then passed to an instance of the Word class, which provides methods to count the number of letters and check if the word is a single word. If the entered word is not a single word, the application outputs an error message and re-prompts the user to enter a word. If the entered word is a single word, the application outputs the number of letters in the word.
相关推荐
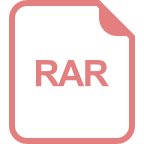
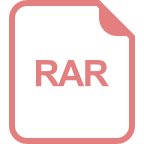
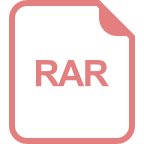














