模拟退火算法python
时间: 2023-06-12 17:07:35 浏览: 92
模拟退火算法(Simulated Annealing,SA)是一种通用概率演算法,用来在一个大的搜寻空间内找寻函数的最优解。下面是一个简单的Python实现:
```python
import math
import random
# 目标函数
def objective_function(x):
return math.sin(10*x) * x + math.cos(2*x) * x
# 状态转移函数:随机生成下一个状态
def get_neighbor(x, step=0.1):
return x + random.uniform(-step, step)
# 计算概率
def acceptance_probability(cost, new_cost, temperature):
if new_cost < cost:
return 1.0
else:
return math.exp((cost - new_cost) / temperature)
# 模拟退火算法
def simulated_annealing(initial_solution, objective_function, get_neighbor, acceptance_probability, temperature=10000, cooling_rate=0.95, stopping_temperature=1e-8):
current_solution = initial_solution
current_cost = objective_function(current_solution)
best_solution = current_solution
best_cost = current_cost
while temperature > stopping_temperature:
for i in range(100):
new_solution = get_neighbor(current_solution)
new_cost = objective_function(new_solution)
ap = acceptance_probability(current_cost, new_cost, temperature)
if ap > random.random():
current_solution = new_solution
current_cost = new_cost
if current_cost < best_cost:
best_solution = current_solution
best_cost = current_cost
temperature *= cooling_rate
return best_solution, best_cost
# 测试
initial_solution = random.uniform(-10, 10)
best_solution, best_cost = simulated_annealing(initial_solution, objective_function, get_neighbor, acceptance_probability)
print("Best solution: {:.4f}".format(best_solution))
print("Best cost: {:.4f}".format(best_cost))
```
在以上代码中,`objective_function`是目标函数,`get_neighbor`是状态转移函数,`acceptance_probability`用于计算接受新解的概率。在`simulated_annealing`函数中,我们通过不断降温来寻找最优解。最后,我们输出最优解和最优解的目标函数值。
阅读全文
相关推荐
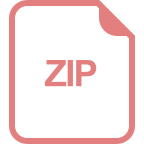


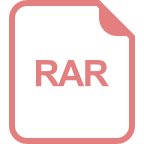









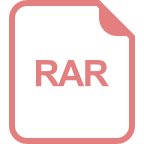