Mapreduce 求各个部门最高工资的员工姓名,用java ,不调用get方法
时间: 2024-02-23 07:58:54 浏览: 73
可以使用MapReduce实现该功能,具体实现步骤如下:
1. Map阶段:将输入的员工信息按照部门编号作为key,员工姓名和工资作为value进行映射。
```java
public static class Map extends Mapper<LongWritable, Text, Text, Text> {
private Text outKey = new Text();
private Text outValue = new Text();
@Override
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
String deptNo = fields[0];
String empName = fields[1];
String salary = fields[2];
outKey.set(deptNo);
outValue.set(empName + ":" + salary);
context.write(outKey, outValue);
}
}
```
2. Reduce阶段:对于每个部门,遍历其所有员工的工资,找出最高工资,并将其对应的员工姓名输出。
```java
public static class Reduce extends Reducer<Text, Text, Text, Text> {
private Text outValue = new Text();
@Override
protected void reduce(Text key, Iterable<Text> values, Context context) throws IOException, InterruptedException {
String maxSalaryEmpName = "";
int maxSalary = Integer.MIN_VALUE;
for (Text value : values) {
String[] fields = value.toString().split(":");
String empName = fields[0];
int salary = Integer.parseInt(fields[1]);
if (salary > maxSalary) {
maxSalary = salary;
maxSalaryEmpName = empName;
}
}
outValue.set(maxSalaryEmpName);
context.write(key, outValue);
}
}
```
3. Driver阶段:设置MapReduce的输入路径、输出路径、Mapper、Reducer等信息,并提交作业。
```java
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "max salary by department");
job.setJarByClass(MaxSalaryByDepartment.class);
job.setMapperClass(Map.class);
job.setReducerClass(Reduce.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(Text.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
```
这样就可以通过MapReduce求出各个部门最高工资的员工姓名了,而且不需要调用get方法。
阅读全文
相关推荐
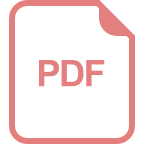

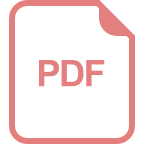







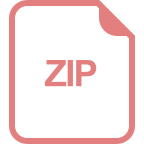
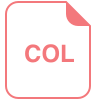
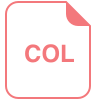
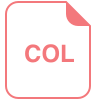


