用C++实现 扫雷游戏
时间: 2023-12-05 12:04:49 浏览: 184
下面是一个用C++实现的扫雷游戏,具体实现思路是使用二维数组来表示扫雷游戏的格子,其中0表示空格,1表示有地雷的格子,2表示已经翻开的格子,3表示标记为地雷的格子。在游戏过程中,使用递归算法来实现翻开空格的效果,同时需要判断游戏是否结束。
```cpp
#include <iostream>
#include <vector>
#include <ctime>
#include <cstdlib>
using namespace std;
// 定义常量
const int BOARD_SIZE = 10; // 扫雷游戏的大小
const int MINE_NUM = 15; // 地雷的数量
// 定义二维数组表示扫雷游戏的格子
int board[BOARD_SIZE][BOARD_SIZE];
// 在指定位置放置地雷
void placeMine(int x, int y) {
board[x][y] = 1;
}
// 随机放置地雷
void placeMines() {
srand(time(nullptr));
int count = 0;
while (count < MINE_NUM) {
int x = rand() % BOARD_SIZE;
int y = rand() % BOARD_SIZE;
if (board[x][y] != 1) {
placeMine(x, y);
count++;
}
}
}
// 计算指定位置周围地雷数量
int countMines(int x, int y) {
int count = 0;
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
int nx = x + i;
int ny = y + j;
if (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE && board[nx][ny] == 1) {
count++;
}
}
}
return count;
}
// 打印游戏面板
void printBoard(bool showMine) {
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (board[i][j] == 2) {
cout << countMines(i, j) << " ";
} else if (board[i][j] == 3) {
cout << "F ";
} else if (showMine && board[i][j] == 1) {
cout << "* ";
} else {
cout << "- ";
}
}
cout << endl;
}
}
// 翻开指定位置的格子
bool click(int x, int y) {
if (board[x][y] == 1) {
return false;
} else if (board[x][y] == 2) {
return true;
} else {
board[x][y] = 2;
if (countMines(x, y) == 0) {
for (int i = -1; i <= 1; ++i) {
for (int j = -1; j <= 1; ++j) {
int nx = x + i;
int ny = y + j;
if (nx >= 0 && nx < BOARD_SIZE && ny >= 0 && ny < BOARD_SIZE) {
click(nx, ny);
}
}
}
}
return true;
}
}
// 标记指定位置的格子
void mark(int x, int y) {
if (board[x][y] == 3) {
board[x][y] = 0;
} else {
board[x][y] = 3;
}
}
// 判断游戏是否结束
bool isGameOver() {
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
if (board[i][j] == 2) {
continue;
} else if (board[i][j] == 1) {
continue;
} else {
return false;
}
}
}
return true;
}
int main() {
// 初始化游戏面板
for (int i = 0; i < BOARD_SIZE; ++i) {
for (int j = 0; j < BOARD_SIZE; ++j) {
board[i][j] = 0;
}
}
placeMines();
// 开始游戏
bool gameOver = false;
while (!gameOver) {
printBoard(false);
cout << "请输入坐标和操作(x y c/m):";
int x, y;
char op;
cin >> x >> y >> op;
if (op == 'm') {
mark(x, y);
} else if (op == 'c') {
if (!click(x, y)) {
gameOver = true;
printBoard(true);
cout << "游戏结束,你输了!" << endl;
} else if (isGameOver()) {
gameOver = true;
printBoard(true);
cout << "恭喜你,你赢了!" << endl;
}
}
}
return 0;
}
```
该程序可以实现一个简单的扫雷游戏,支持放置地雷、随机放置地雷、计算周围地雷数量、打印游戏面板、翻开格子、标记格子、判断游戏是否结束等操作。需要注意的是,由于该程序使用了递归算法来实现翻开空格的效果,可能会出现栈溢出的情况,因此需要设置递归深度的限制。
阅读全文
相关推荐
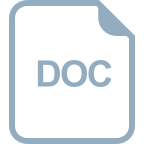
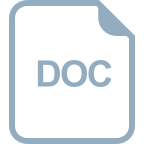
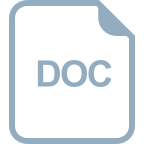
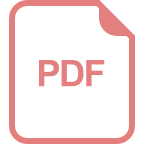
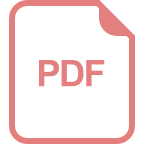
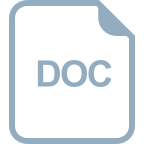
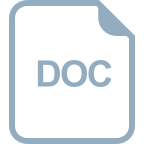
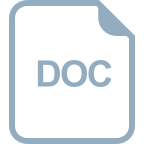
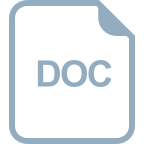
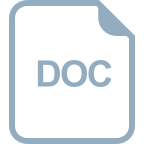

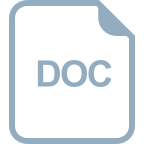
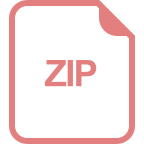