编程实现程序:(1)计算n!并打印结果;(2)输入n值,打印从1-n之间所有整数的阶乘;(3)计算1!+2!+3!+…+n!并打印结果;(4)显示功能列表清单; 以上功能通过函数实现,由一个主函数内按功能清单列表选择调用,用C语言编写改程序
时间: 2024-10-11 07:08:23 浏览: 95
在C语言中,我们可以创建四个不同的函数分别完成这些任务,并在一个主函数中作为选项供用户选择调用。以下是这些功能的简单实现:
```c
#include <stdio.h>
// 计算阶乘
unsigned long long factorial(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * factorial(n - 1);
}
// 打印从1到n的每个整数的阶乘
void printFactorials(int n) {
for (int i = 1; i <= n; i++) {
printf("%d! = %llu\n", i, factorial(i));
}
}
// 计算并打印1! + 2! + ... + n!
long long sumOfFactorials(int n) {
long long result = 0;
for (int i = 1; i <= n; i++) {
result += factorial(i);
}
printf("Sum of factorials from 1 to %d: %lld\n", n, result);
}
// 显示功能列表
void displayFunctionList() {
printf("\nFunction List:\n");
printf("1. Calculate and print individual factorials\n");
printf("2. Sum of factorials up to a given number\n");
printf("3. Exit program\n");
}
// 主函数
int main() {
int choice;
do {
printf("\nPlease choose an option:\n");
scanf("%d", &choice);
switch(choice) {
case 1:
int input_n;
printf("Enter the number: ");
scanf("%d", &input_n);
printFactorials(input_n);
break;
case 2:
int input_n_2;
printf("Enter the upper limit: ");
scanf("%d", &input_n_2);
sumOfFactorials(input_n_2);
break;
case 3:
printf("Exiting the program...\n");
break;
default:
printf("Invalid choice. Please try again.\n");
}
} while (choice != 3); // Continue until user chooses '3' to exit
return 0;
}
```
这个程序会循环接收用户的输入,然后根据用户的选择调用相应的函数。如果你想要运行这个程序,你需要将它复制到一个`.c`文件中,然后通过编译器如gcc来编译并运行。
阅读全文
相关推荐
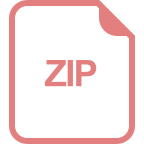
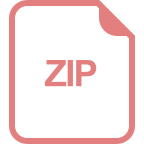
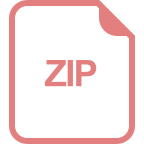

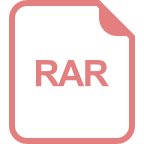

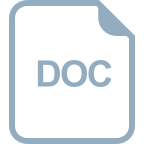










