H.264 封装mp4源码
时间: 2023-12-06 15:03:20 浏览: 34
H.264 封装 mp4 的源码可以使用开源库 ffmpeg 来实现。以下是一个示例代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdint.h>
#include <time.h>
#include <libavcodec/avcodec.h>
#include <libavformat/avformat.h>
#include <libavutil/opt.h>
#include <libswscale/swscale.h>
int main(int argc, char *argv[]) {
AVFormatContext *fmt_ctx = NULL;
AVOutputFormat *out_fmt = NULL;
AVStream *video_stream = NULL;
AVCodecContext *codec_ctx = NULL;
AVCodec *codec = NULL;
AVPacket pkt = { 0 };
if (argc != 3) {
printf("Usage: %s <input_file> <output_file>\n", argv[0]);
return 1;
}
av_register_all();
if (avformat_open_input(&fmt_ctx, argv[1], NULL, NULL) < 0) {
printf("Error: Failed to open input file %s\n", argv[1]);
return 1;
}
if (avformat_find_stream_info(fmt_ctx, NULL) < 0) {
printf("Error: Failed to find stream information\n");
return 1;
}
for (int i = 0; i < fmt_ctx->nb_streams; i++) {
AVStream *stream = fmt_ctx->streams[i];
AVCodecParameters *codecpar = stream->codecpar;
if (codecpar->codec_type == AVMEDIA_TYPE_VIDEO) {
codec = avcodec_find_decoder(codecpar->codec_id);
if (!codec) {
printf("Error: Failed to find decoder for video stream\n");
return 1;
}
codec_ctx = avcodec_alloc_context3(codec);
if (!codec_ctx) {
printf("Error: Failed to allocate codec context\n");
return 1;
}
if (avcodec_parameters_to_context(codec_ctx, codecpar) < 0) {
printf("Error: Failed to copy codec parameters to context\n");
return 1;
}
if (avcodec_open2(codec_ctx, codec, NULL) < 0) {
printf("Error: Failed to open codec\n");
return 1;
}
video_stream = avformat_new_stream(fmt_ctx, codec);
if (!video_stream) {
printf("Error: Failed to create new stream\n");
return 1;
}
if (avcodec_parameters_copy(video_stream->codecpar, codecpar) < 0) {
printf("Error: Failed to copy codec parameters\n");
return 1;
}
out_fmt = av_guess_format(NULL, argv[2], NULL);
if (!out_fmt) {
printf("Error: Failed to guess output format\n");
return 1;
}
if (avio_open(&fmt_ctx->pb, argv[2], AVIO_FLAG_WRITE) < 0) {
printf("Error: Failed to open output file\n");
return 1;
}
if (avformat_write_header(fmt_ctx, NULL) < 0) {
printf("Error: Failed to write format header\n");
return 1;
}
}
}
if (!video_stream) {
printf("Error: Failed to find video stream\n");
return 1;
}
while (av_read_frame(fmt_ctx, &pkt) == 0) {
if (pkt.stream_index == video_stream->index) {
if (av_write_frame(fmt_ctx, &pkt) < 0) {
printf("Error: Failed to write frame\n");
return 1;
}
}
av_packet_unref(&pkt);
}
if (av_write_trailer(fmt_ctx) < 0) {
printf("Error: Failed to write trailer\n");
return 1;
}
avcodec_free_context(&codec_ctx);
avformat_close_input(&fmt_ctx);
avformat_free_context(fmt_ctx);
return 0;
}
```
这个代码可以打开一个 H.264 视频文件,封装成 MP4 格式的文件并输出到指定的输出文件中。你可以根据实际情况修改代码中的输入文件和输出文件路径。
相关推荐
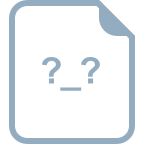














