请用proto传输bytes类型举例,用C++实现
时间: 2024-03-06 12:47:27 浏览: 19
好的,下面是一个使用 Protobuf 传输 `bytes` 类型的例子,实现语言为 C++:
首先,定义一个 `.proto` 文件,例如:
```protobuf
syntax = "proto3";
message ExampleMessage {
bytes data = 1;
}
```
上述代码定义了一个名为 `ExampleMessage` 的消息类型,包含一个 `bytes` 类型的字段 `data`。
接下来,使用 `protoc` 工具编译 `.proto` 文件,生成相应的 C++ 代码。假设生成的文件为 `example.pb.cc` 和 `example.pb.h`。
然后,可以使用以下代码创建一个 `ExampleMessage` 对象,将一个 `std::string` 转换成 `bytes` 类型,并将其赋值给 `data` 字段:
```c++
#include <iostream>
#include <string>
#include "example.pb.h"
int main() {
ExampleMessage message;
std::string str = "hello world";
message.mutable_data()->assign(str);
// 序列化为字节流
std::string output = message.SerializeAsString();
// 反序列化为对象
ExampleMessage deserialized_message;
deserialized_message.ParseFromString(output);
std::cout << deserialized_message.data() << std::endl;
return 0;
}
```
上述代码中,`mutable_data()` 方法返回一个 `std::string` 对象的指针,可以通过该指针修改 `data` 字段的值。`SerializeAsString()` 方法将 `ExampleMessage` 对象序列化为一个字节流,`ParseFromString()` 方法将字节流反序列化为一个对象。
注意,`bytes` 类型的字段在序列化时不会进行编码,直接将字节流写入到输出流中。因此,在传输时需要注意字节序问题。
相关推荐
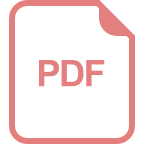
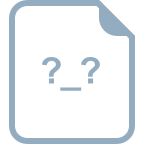














