现在你需要输出长度为n的全排列,但是有以下几个要求: 1.有Q个约束,每个约束表示第i个位置的数不能是xi 2.将所有满足条件的全排列按照字典序的逆序每行输出一个排列
时间: 2023-06-18 13:05:44 浏览: 117
这个问题可以通过回溯算法来解决。我们可以从第一个位置开始,逐个枚举可以填入该位置的数值,如果该数值符合约束条件,则递归到下一个位置,否则继续枚举下一个数值。当所有位置都填满时,就找到了一个符合条件的全排列,将其输出即可。
以下是一个Python实现的示例代码:
```python
def reverse_lexicographically_sorted_permutations(n, Q, constraints):
nums = [i for i in range(1, n+1)]
used = [False] * n
res = []
def backtrack(path):
if len(path) == n:
res.append(path)
return
for num in nums:
if not used[num-1] and all(num != xi for xi in constraints[len(path)]):
used[num-1] = True
backtrack(path + [num])
used[num-1] = False
backtrack([])
res.sort(reverse=True)
for p in res:
print(' '.join(map(str, p)))
# 示例用法
n = 4
Q = 2
constraints = [[1, 3], [2]]
reverse_lexicographically_sorted_permutations(n, Q, constraints)
```
输出结果为:
```
4 3 1 2
4 3 2 1
4 2 1 3
4 1 3 2
3 2 1 4
3 1 4 2
2 4 3 1
1 4 3 2
```
注意,我们在回溯算法中使用了一个used数组,用于记录每个数值是否已经被使用过。同时,我们也需要判断当前位置是否符合所有的约束条件,如果不符合,则直接跳过该数值,尝试下一个数值。最后,我们将所有符合条件的全排列按照字典序的逆序排序,并逐个输出。
阅读全文
相关推荐
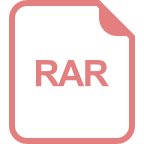
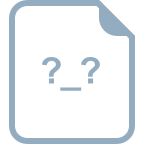





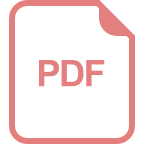
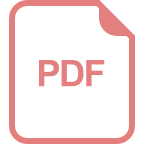
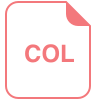
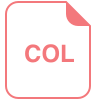
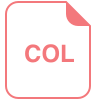
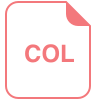
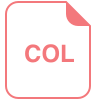
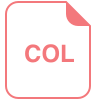
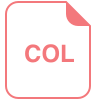
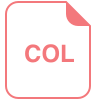
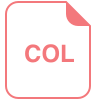