struct Student { char no[20]; char name[50]; float score; };void swp(Student &s1, Student &s2){ }swp函数完成结构体的交换怎么写
时间: 2024-09-12 16:02:56 浏览: 34
在C++中,`struct Student`定义了一个结构体,包含了一个学号字符串`no`,一个姓名字符串`name`和一个成绩浮点数`score`。要编写一个`swp`函数来交换两个`Student`结构体变量的内容,你可以使用C++标准库中的`std::swap`函数,或者手动编写交换逻辑。下面给出两种方式的示例代码:
使用`std::swap`函数的示例:
```cpp
#include <algorithm> // 引入算法库以使用swap
struct Student {
char no[20];
char name[50];
float score;
};
void swp(Student &s1, Student &s2) {
std::swap(s1.no, s2.no);
std::swap(s1.name, s2.name);
std::swap(s1.score, s2.score);
}
```
手动编写交换逻辑的示例:
```cpp
#include <cstring> // 引入字符串处理库以使用strcpy
struct Student {
char no[20];
char name[50];
float score;
};
void swp(Student &s1, Student &s2) {
// 由于字符串数组不能直接使用swap,需要逐字节复制
char temp_no[20];
char temp_name[50];
// 交换学号
strcpy(temp_no, s1.no);
strcpy(s1.no, s2.no);
strcpy(s2.no, temp_no);
// 交换姓名
strcpy(temp_name, s1.name);
strcpy(s1.name, s2.name);
strcpy(s2.name, temp_name);
// 交换成绩,直接使用swap
std::swap(s1.score, s2.score);
}
```
注意:当使用`std::swap`函数时,如果`no`和`name`是字符数组,`std::swap`会逐字节交换数据,这可能会导致字符串的结束符`'\0'`位置也交换,从而破坏字符串。因此在实际情况中,建议使用`strcpy`或`std::string`来处理字符串交换,如第二种示例所示。
阅读全文
相关推荐
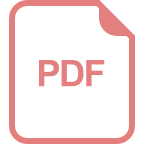
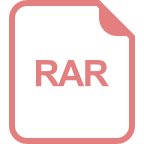
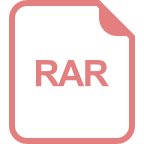



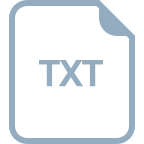










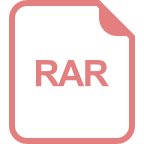
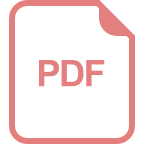