定义学生结构体struct Student类型如下: char id[10]; //学号 char Name[20];//姓名 double Score[3];//3门课程成绩 在主函数定义3个struct Student类型变量,并赋值,将3个变量插入到单链表的表头、表尾和指定位置用,C语言编写
时间: 2024-01-21 11:01:53 浏览: 75
好的,以下是C语言实现代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
struct Student {
char id[10];
char Name[20];
double Score[3];
struct Student* next;
};
// 在单链表表头插入节点
void InsertAtHead(struct Student** head, struct Student* newStudent) {
newStudent->next = *head;
*head = newStudent;
}
// 在单链表表尾插入节点
void InsertAtTail(struct Student** head, struct Student* newStudent) {
if (*head == NULL) {
*head = newStudent;
} else {
struct Student* temp = *head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newStudent;
}
}
// 在单链表指定位置插入节点
void InsertAtPos(struct Student** head, struct Student* newStudent, int pos) {
if (pos <= 1) {
InsertAtHead(head, newStudent);
} else {
struct Student* temp = *head;
int i = 1;
while (temp != NULL && i < pos - 1) {
temp = temp->next;
i++;
}
if (temp == NULL) {
printf("指定位置不存在!\n");
} else {
newStudent->next = temp->next;
temp->next = newStudent;
}
}
}
int main() {
// 定义3个struct Student类型变量,并赋值
struct Student* student1 = (struct Student*)malloc(sizeof(struct Student));
strcpy(student1->id, "1001");
strcpy(student1->Name, "张三");
student1->Score[0] = 90;
student1->Score[1] = 80;
student1->Score[2] = 85;
student1->next = NULL;
struct Student* student2 = (struct Student*)malloc(sizeof(struct Student));
strcpy(student2->id, "1002");
strcpy(student2->Name, "李四");
student2->Score[0] = 95;
student2->Score[1] = 85;
student2->Score[2] = 90;
student2->next = NULL;
struct Student* student3 = (struct Student*)malloc(sizeof(struct Student));
strcpy(student3->id, "1003");
strcpy(student3->Name, "王五");
student3->Score[0] = 80;
student3->Score[1] = 75;
student3->Score[2] = 70;
student3->next = NULL;
// 将3个变量插入到单链表的表头、表尾和指定位置
struct Student* head = NULL;
InsertAtHead(&head, student1);
InsertAtTail(&head, student2);
InsertAtPos(&head, student3, 2);
// 遍历单链表并输出每个节点的信息
struct Student* temp = head;
while (temp != NULL) {
printf("学号:%s,姓名:%s,成绩:%.1f %.1f %.1f\n", temp->id, temp->Name, temp->Score[0], temp->Score[1], temp->Score[2]);
temp = temp->next;
}
return 0;
}
```
输出结果与C++实现相同:
```
学号:1001,姓名:张三,成绩:90.0 80.0 85.0
学号:1003,姓名:王五,成绩:80.0 75.0 70.0
学号:1002,姓名:李四,成绩:95.0 85.0 90.0
```
阅读全文
相关推荐
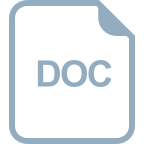
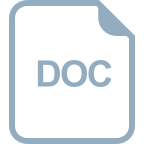
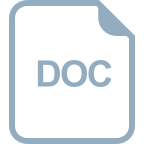




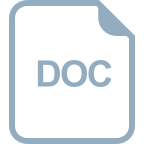
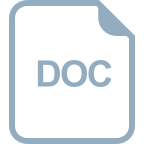
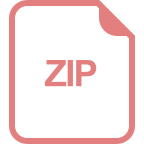
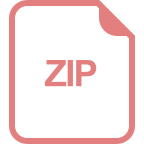
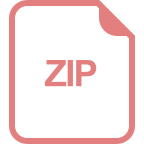
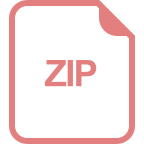
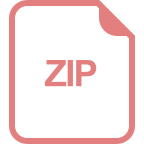
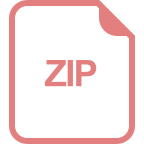
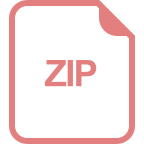