C语言 简单的CRC 代码
时间: 2024-11-09 19:22:57 浏览: 18
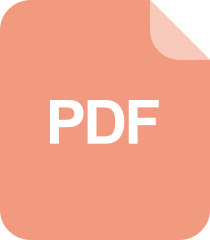
CRC4的C语言实现代码
在C语言中,计算CRC (Cyclic Redundancy Check) 通常涉及到位操作和异或(XOR)运算。这里是一个简单的CRC-8算法的实现示例:
```c
#include <stdio.h>
// CRC-8 polynomial (0x7)
#define CRC_POLY 0x7
// Generate a CRC byte for a given input data and initial value
unsigned char crc8_calculate(unsigned char data, unsigned char init_val) {
static const unsigned char poly_table[256] = {
0x00, 0x07, 0x0e, 0x09, 0x1c, 0x1b, 0x12, 0x15,
... // Fill the rest of the table with polynomial division results
};
unsigned char crc = init_val ^ data;
for (int i = 0; i < sizeof(data); ++i) {
crc = poly_table[(crc ^ data[i]) & 0xff];
}
return crc;
}
int main() {
unsigned char data[] = {0x43, 0x21, 0x0a, 0x1f};
unsigned char init_val = 0x00; // Initial CRC value, usually zero
unsigned char result = crc8_calculate(data, init_val);
printf("CRC-8 check sum: %02X\n", result);
return 0;
}
```
这个例子中,`poly_table`是一个预计算的表格,用于进行位级的异或操作。CRC校验过程会不断将当前的CRC值与输入数据进行异或,然后根据CRC POLY(在这个例子中是0x7)进行模2除法。
阅读全文
相关推荐
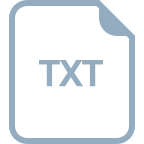
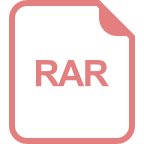



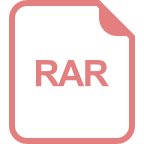
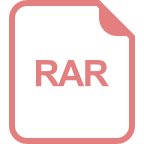
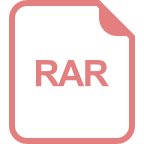
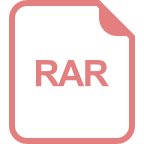
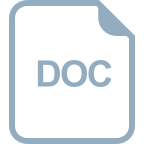
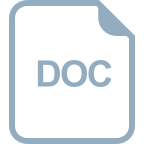
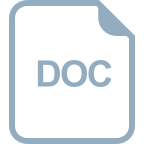



