设计一个verilog代码fpga实现电子琴学习功能,led灯显示对应的音阶序号,按键分别对应发出七个音阶,如果按错或者超过一定时间,就重头开始,芯片为ep4ce6e22c8
时间: 2024-03-22 12:39:28 浏览: 56
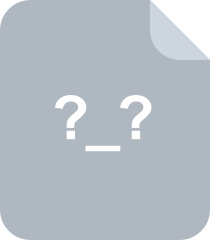
用Verilog实现的电子琴
为了实现您所描述的电子琴学习功能,需要编写一个Verilog代码来控制FPGA。以下是基本的代码框架:
```
module electronic_piano(
input clk, // 时钟信号
input rst, // 复位信号
input [6:0] keys, // 按键输入
output reg [6:0] leds, // LED灯输出
output reg dac_out // 音频输出
);
// 定义状态机状态
typedef enum logic [2:0] {
IDLE, // 空闲状态
PLAYING, // 播放状态
ERROR // 错误状态
} state_t;
// 定义FPGA时钟频率(单位MHz)
parameter fpga_clk_freq = 50;
// 定义按键扫描周期(单位ms)
parameter scan_period = 20;
// 定义音阶序列
logic [6:0] note_sequence [0:6] = '{7'b1000000, 7'b1100000, 7'b1110000, 7'b1111000, 7'b1111100, 7'b1111110, 7'b1111111};
// 定义状态机变量
state_t state;
logic [2:0] note_index;
logic [6:0] current_note;
logic [31:0] key_scan_timer;
// 定义按键扫描计数器
logic [2:0] scan_counter;
// 按键扫描模块
always @(posedge clk) begin
if (rst) begin
key_scan_timer <= 0;
scan_counter <= 0;
end else begin
key_scan_timer <= key_scan_timer + 1;
if (key_scan_timer >= fpga_clk_freq * scan_period / 1000) begin
key_scan_timer <= 0;
scan_counter <= scan_counter + 1;
if (scan_counter >= 7) begin
scan_counter <= 0;
end
end
end
end
// 状态机模块
always @(posedge clk) begin
if (rst) begin
state <= IDLE;
note_index <= 0;
current_note <= 7'b0000000;
leds <= 7'b0000000;
dac_out <= 16'h0000;
end else begin
case (state)
IDLE: begin
// 空闲状态
if (keys[scan_counter] == 1'b1) begin
note_index <= 0;
current_note <= note_sequence[note_index];
leds <= note_index;
dac_out <= current_note;
state <= PLAYING;
end
end
PLAYING: begin
// 播放状态
if (keys[scan_counter] == 1'b0) begin
state <= ERROR;
end else if (note_index == 6) begin
state <= IDLE;
note_index <= 0;
current_note <= 7'b0000000;
leds <= 7'b0000000;
dac_out <= 16'h0000;
end else begin
note_index <= note_index + 1;
current_note <= note_sequence[note_index];
leds <= note_index;
dac_out <= current_note;
end
end
ERROR: begin
// 错误状态
state <= IDLE;
note_index <= 0;
current_note <= 7'b0000000;
leds <= 7'b0000000;
dac_out <= 16'h0000;
end
endcase
end
end
endmodule
```
在这个代码中,首先定义了输入输出端口,包括时钟信号、复位信号、按键输入、LED灯输出和音频输出。然后定义了状态机的状态类型和变量,以及按键扫描计数器和计时器。在按键扫描模块中,通过计时器控制按键的扫描周期。在状态机模块中,根据不同的状态进行相应的状态转移和操作,包括空闲状态、播放状态和错误状态。在空闲状态下,如果检测到有按键按下,则进入播放状态,并输出对应的音阶和LED灯序号。在播放状态下,如果检测到按键按错或者播放完所有的音阶,则进入错误状态或者回到空闲状态。在错误状态下,重新回到空闲状态并清除状态机变量。最后,在主模块中实例化状态机,并连接输入输出端口。
希望这个代码框架能够帮助您完成您的项目!
阅读全文
相关推荐
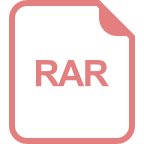

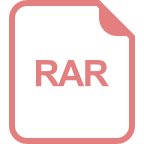
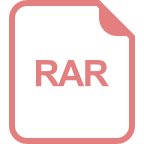
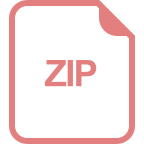
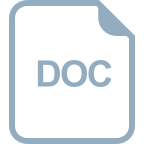
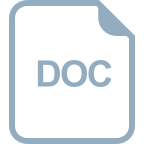
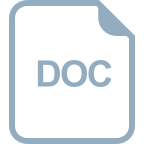
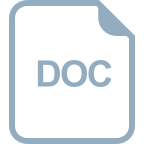





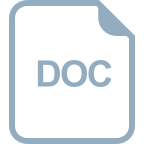
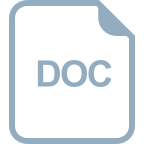
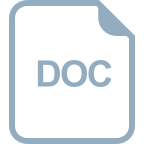