from typing import List, Dict def dfs(index: int, must: str, mustCharIdx: Dict[str, List[int]], path: List[int], ans: List[int]) -> None: if len(path) == len(must): dis = path[0] for i in range(1, len(path)): dis += abs(path[i] - path[i - 1]) ans[0] = min(ans[0], dis) return for i in range(index, len(must)): c = must[i] idxs = mustCharIdx[c] for idx in idxs: path.append(idx) dfs(i + 1, must, mustCharIdx, path, ans) path.pop() if __name__ == '__main__': all_str = input() must_str = input() mustCharIdx = {} mustChar = set(must_str) for i in range(len(all_str)): c = all_str[i] if c in mustChar: if c not in mustCharIdx: mustCharIdx[c] = [] mustCharIdx[c].append(i) ans = [float("inf")] path = [] dfs(0, must_str, mustCharIdx, path, ans) print(ans[0])上述代码中,为什么要path.pop()
时间: 2024-02-14 10:26:48 浏览: 58
在给定的代码中,`path.pop()`被用于回溯操作。在回溯法中,当我们在搜索过程中尝试了一个不符合条件的路径或者找到了一个解后,我们需要返回到上一步进行新的尝试。
在这段代码中,当递归调用`dfs(i + 1, must, mustCharIdx, path, ans)`返回后,我们需要将当前尝试的节点从路径中移除,以便能够尝试其他的节点。这样做可以确保在回溯到上一个节点时,路径状态恢复到之前的状态。
简单来说,`path.pop()`用于在搜索过程中回退到上一步,以便继续搜索其他可能的路径。这是回溯法中常见的操作之一。
希望这样解答了你的疑问!如果还有其他问题,请随时提问。
相关问题
from typing import List, Dict
这是 Python 3 中用于声明函数参数类型和返回值类型的语法。`List` 表示一个列表,`Dict` 表示一个字典。在声明时,需要在参数名后面用冒号 `:` 指定参数类型,如果需要多个参数,则用逗号 `,` 分隔。如果函数有返回值,则需要在参数声明后面用箭头 `->` 指定返回值类型,如果没有返回值则可以省略。
例如,下面的代码声明了一个名为 `count_words` 的函数,该函数接收一个字符串列表作为参数,返回一个字典,其中键为字符串,值为该字符串在列表中出现的次数:
```python
def count_words(words: List[str]) -> Dict[str, int]:
counts = {}
for word in words:
counts[word] = counts.get(word, 0) + 1
return counts
```
from typing import Dict
`from typing import Dict` 是用来导入 Python 的 typing 模块中的 Dict 类型的定义。typing 模块是 Python 3.5+ 引入的一个模块,用于提供类型提示和类型注解的支持。Dict 是用来表示字典类型的注解,它指定了字典键和值的类型。例如,`Dict[str, int]` 表示一个键是字符串类型,值是整数类型的字典。通过使用 typing 模块中的类型注解,可以提高代码的可读性和维护性,并在静态类型检查工具(如mypy)中进行类型检查。
阅读全文
相关推荐
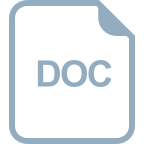
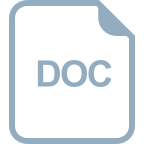
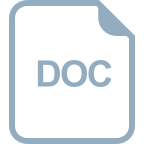
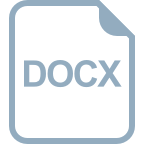













