用C语言写一个在lcd上触摸滚动条,lcd文本显示翻页的例程
时间: 2023-05-14 09:06:39 浏览: 95
非常感谢您的提问!以下是一个简单的用C语言编写的在LCD上触摸滚动条和文本翻页的例程:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <stdbool.h>
// 定义LCD的宽度和高度
#define LCD_WIDTH 480
#define LCD_HEIGHT 320
// 定义滚动条的宽度和高度
#define SCROLLBAR_WIDTH 10
#define SCROLLBAR_HEIGHT 100
// 定义文本框的宽度和高度
#define TEXTBOX_WIDTH 400
#define TEXTBOX_HEIGHT 200
// 定义文本框中每行文本的最大长度
#define MAX_LINE_LENGTH 50
// 定义文本框中每页最多显示的行数
#define MAX_LINES_PER_PAGE 10
// 定义文本框中的文本
const char *text = "这是一个在LCD上触摸滚动条和文本翻页的例程,用C语言编写。"
"它可以在LCD上显示一个文本框和一个滚动条,当文本框中的文本超过"
"一屏时,可以通过滚动条滚动文本框中的文本。";
// 定义滚动条的位置和文本框的位置
int scrollbar_x = LCD_WIDTH - SCROLLBAR_WIDTH - 10;
int scrollbar_y = 10;
int textbox_x = 10;
int textbox_y = 10;
// 定义文本框中的当前页和总页数
int current_page = 1;
int total_pages = 1;
// 定义滚动条是否被按下的标志
bool scrollbar_pressed = false;
// 定义函数:绘制滚动条
void draw_scrollbar()
{
// 计算滚动条的位置和长度
int scrollbar_length = (int)((float)SCROLLBAR_HEIGHT / (float)total_pages);
int scrollbar_pos = scrollbar_y + (int)(((float)current_page - 1.0f) / ((float)total_pages - 1.0f) * (float)(SCROLLBAR_HEIGHT - scrollbar_length));
// 绘制滚动条的背景
// ...
// 绘制滚动条的滑块
// ...
// 绘制滚动条的边框
// ...
}
// 定义函数:绘制文本框
void draw_textbox()
{
// 计算文本框中每页最多显示的行数
int max_lines_per_page = (int)((float)TEXTBOX_HEIGHT / (float)FONT_HEIGHT);
// 计算文本框中每行文本的最大长度
int max_line_length = (int)((float)TEXTBOX_WIDTH / (float)FONT_WIDTH);
// 计算文本框中的总页数
total_pages = strlen(text) / (max_lines_per_page * max_line_length) + 1;
// 计算文本框中当前页的起始位置和结束位置
int start_pos = (current_page - 1) * max_lines_per_page * max_line_length;
int end_pos = start_pos + max_lines_per_page * max_line_length;
// 如果结束位置超过了文本的总长度,则将结束位置设置为文本的总长度
if (end_pos > strlen(text))
{
end_pos = strlen(text);
}
// 绘制文本框的背景
// ...
// 绘制文本框中的文本
int line_count = 0;
int line_pos = 0;
char line[MAX_LINE_LENGTH + 1];
for (int i = start_pos; i < end_pos; i++)
{
if (text[i] == '\n')
{
line[line_pos] = '\0';
draw_text(line, textbox_x, textbox_y + line_count * FONT_HEIGHT);
line_count++;
line_pos = 0;
}
else
{
line[line_pos] = text[i];
line_pos++;
if (line_pos >= MAX_LINE_LENGTH)
{
line[line_pos] = '\0';
draw_text(line, textbox_x, textbox_y + line_count * FONT_HEIGHT);
line_count++;
line_pos = 0;
}
}
}
if (line_pos > 0)
{
line[line_pos] = '\0';
draw_text(line, textbox_x, textbox_y + line_count * FONT_HEIGHT);
line_count++;
}
// 绘制文本框的边框
// ...
}
// 定义函数:处理触摸事件
void handle_touch_event(int x, int y, int event_type)
{
// 判断触摸事件是否在滚动条上
if (x >= scrollbar_x && x < scrollbar_x + SCROLLBAR_WIDTH &&
y >= scrollbar_y && y < scrollbar_y + SCROLLBAR_HEIGHT)
{
// 如果是按下事件,则将滚动条的按下标志设置为true
if (event_type == TOUCH_EVENT_PRESS)
{
scrollbar_pressed = true;
}
// 如果是移动事件,则根据触摸位置计算当前页数
else if (event_type == TOUCH_EVENT_MOVE && scrollbar_pressed)
{
float pos = (float)(y - scrollbar_y) / (float)SCROLLBAR_HEIGHT;
current_page = (int)(pos * (float)(total_pages - 1)) + 1;
if (current_page < 1)
{
current_page = 1;
}
if (current_page > total_pages)
{
current_page = total_pages;
}
}
// 如果是释放事件,则将滚动条的按下标志设置为false
else if (event_type == TOUCH_EVENT_RELEASE)
{
scrollbar_pressed = false;
}
}
}
// 主函数
int main()
{
// 初始化LCD
// ...
// 初始化触摸屏
// ...
// 绘制滚动条和文本框
draw_scrollbar();
draw_textbox();
// 进入主循环
while (true)
{
// 处理触摸事件
int x, y, event_type;
if (get_touch_event(&x, &y, &event_type))
{
handle_touch_event(x, y, event_type);
}
// 绘制滚动条和文本框
draw_scrollbar();
draw_textbox();
// 刷新LCD
// ...
}
return 0;
}
```
希望这个例程能够帮助您实现在LCD上触摸滚动条和文本翻页的功能!如果您有任何问题或建议,请随时联系我。
相关推荐
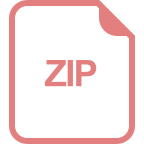














