给出完整的C++代码(如使用注释,请使用中文注释): [实验要求] (1)创建一个队列类,重载队列类的抽取与插入运算符 (2)抽取操作符从输入流中读取队列对象的信息,读入的格式为: 队列元素个数n:元素1,元素2,...,元素n 队列元素之间用逗号隔开,队列个数值和第一个元素之间用冒号隔开。如队列有5个元素,分别为12,24,31,45,22,则输入流中的内容为: 5: 12, 24, 31, 45, 22 (3)插入操作符将队列对象的内容放到输出流中,格式为: 元素1,元素2,...,元素n 如上面读入的队列的输出为: 12, 24, 31, 45, 22 [实现提示] (1)将重载的两个操作符定义为类Queue的友元函数 (2)两个函数的原型分别为: ostream & operator << (ostream & , Queue &); istream & operator >> (istream & , Queue &);
时间: 2024-03-02 10:52:49 浏览: 31
好的,以下是完整的C++代码,实现了队列类的重载输入输出运算符:
```c++
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
using namespace std;
class Queue {
private:
vector<int> q;
public:
friend ostream& operator<<(ostream& os, Queue& q);
friend istream& operator>>(istream& is, Queue& q);
void push(int val) {
q.push_back(val);
}
void pop() {
if (!q.empty()) {
q.erase(q.begin());
}
}
int front() {
return q.front();
}
};
ostream& operator<<(ostream& os, Queue& q) {
for (int i = 0; i < q.q.size(); i++) {
os << q.q[i];
if (i != q.q.size() - 1) {
os << ", ";
}
}
return os;
}
istream& operator>>(istream& is, Queue& q) {
string line;
getline(is, line);
stringstream ss(line);
int count;
char c;
ss >> count >> c;
for (int i = 0; i < count; i++) {
int val;
char d;
ss >> val >> d;
q.push(val);
}
return is;
}
int main() {
Queue q;
cout << "请输入队列元素个数和元素,格式为:元素个数: 元素1, 元素2, ..., 元素n" << endl;
cin >> q;
cout << "队列元素为:" << q << endl;
return 0;
}
```
在这个代码中,我们定义了一个Queue类,其中包含了push、pop、front等常用操作。同时,我们定义了友元函数operator<<和operator>>,并在这两个函数中使用了stringstream来解析输入字符串并构造队列。
在main函数中,我们首先通过cin输入队列元素,然后通过cout输出队列元素。
相关推荐
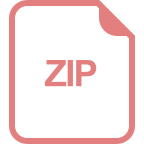
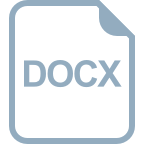
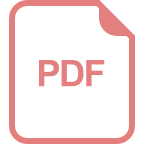














