cgan网络的adam优化器用一个beta和两个beta的区别
时间: 2024-01-30 19:03:31 浏览: 62
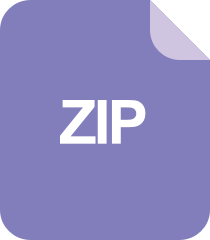
TFM_CGAN:在Keras上建立一个生成对抗网络
CGAN(Conditional Generative Adversarial Networks,有条件生成对抗网络)是一种生成对抗网络,它可以利用条件信息来生成具有特定条件的数据。
在CGAN中,Adam优化器通常使用两个beta参数来控制动量的衰减和梯度平方的衰减。具体来说,Adam优化器有三个重要的参数,分别是learning rate、beta1和beta2。其中,beta1控制动量的衰减,beta2控制梯度平方的衰减。当beta1=0.9,beta2=0.999时,Adam优化器在训练过程中表现良好。
而如果只使用一个beta参数,那么Adam优化器就相当于是带有动量的RMSProp算法。因此,在CGAN中,使用两个beta参数的Adam优化器可以更好地控制动量和梯度平方的衰减,从而提高训练的稳定性和效果。
阅读全文
相关推荐
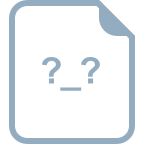
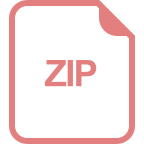















