python五子棋算法
时间: 2023-10-24 14:21:27 浏览: 45
五子棋是一种非常经典的棋类游戏,下面是一个简单的 Python 实现五子棋算法的示例:
```python
import numpy as np
class FiveChess:
def __init__(self, width=15, height=15, win_len=5):
self.width = width
self.height = height
self.win_len = win_len
self.board = np.zeros((height, width))
self.current_player = 1
self.is_over = False
def move(self, x, y):
if self.board[y][x] != 0:
return False
self.board[y][x] = self.current_player
if self.check_win(x, y):
self.is_over = True
print("Player %d wins!" % self.current_player)
return True
self.current_player = 3 - self.current_player
return True
def check_win(self, x, y):
directions = [(0, 1), (1, 0), (1, 1), (1, -1)]
for dx, dy in directions:
count = 1
tx, ty = x, y
while tx + dx >= 0 and tx + dx < self.width and \
ty + dy >= 0 and ty + dy < self.height and \
count < self.win_len and \
self.board[ty + dy][tx + dx] == self.current_player:
count += 1
tx += dx
ty += dy
tx, ty = x, y
while tx - dx >= 0 and tx - dx < self.width and \
ty - dy >= 0 and ty - dy < self.height and \
count < self.win_len and \
self.board[ty - dy][tx - dx] == self.current_player:
count += 1
tx -= dx
ty -= dy
if count == self.win_len:
return True
return False
```
在这个示例中,我们使用了一个二维数组来表示棋盘。我们在初始化时指定了棋盘的大小和获胜所需连续棋子的数量。`move` 方法用于在指定的位置下棋,并且检查是否有一方获胜。`check_win` 方法用于检查是否有一方获胜。在检查时,我们使用了四个方向:水平、垂直和两个对角线。如果在任何一个方向上有足够的连续棋子,则返回 `True`,否则返回 `False`。
相关推荐
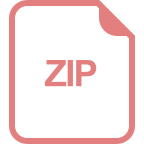














