python 判断五子棋 算法
时间: 2023-11-10 14:29:59 浏览: 37
五子棋的判断算法可以采用连续五个棋子在同一条线上的方式进行判断。具体实现可以通过以下步骤:
1. 遍历棋盘上的每个位置,如果该位置为空则跳过,否则进行以下步骤。
2. 以该位置为起点,向横、竖、左上右下、左下右上四个方向延伸,判断是否有连续五个相同颜色的棋子在同一条线上。
3. 如果有连续五个相同颜色的棋子在同一条线上,则判断该颜色的棋子获胜。
4. 如果所有位置都遍历完了,但没有出现连续五个相同颜色的棋子在同一条线上,则判断为平局。
注意事项:
1. 在判断时需要注意边界问题,避免发生数组越界等错误。
2. 如果棋盘过大,遍历所有位置的计算量会很大,可以考虑采用一些优化算法,如加入启发式搜索等。
3. 还需要考虑其他特殊情况,如死局、长连禁手等,可以根据需要适当调整算法。
相关问题
python判断五子棋算法
五子棋是一种非常经典的棋类游戏,判断五子棋胜负的算法可以通过以下步骤实现:
1. 创建一个二维数组表示棋盘,用0表示空位,1表示黑棋,2表示白棋。
2. 在每次下棋后,判断该位置是否有五子相连。
3. 对于每个位置,可以分别向右、下、右下、左下四个方向进行判断。
4. 如果某个方向上已经有四颗棋子,再放一颗同色棋子时就可以判断胜负。
5. 如果在所有方向上都没有五子相连,就继续下棋,直到棋盘被填满或者出现五子相连。
具体实现可以参考以下代码:
```python
# 创建一个15x15的二维数组表示棋盘
board = [[0] * 15 for i in range(15)]
# 判断某个位置是否能下棋
def can_move(x, y, color):
if x < 0 or x >= 15 or y < 0 or y >= 15 or board[x][y] != 0:
return False
# 如果该位置上下左右四个方向已经有四颗同色棋子,则可以下棋
dx = [0, 1, 1, 1]
dy = [1, 0, 1, -1]
for i in range(4):
count = 1
for j in range(1, 5):
tx = x + j * dx[i]
ty = y + j * dy[i]
if tx < 0 or tx >= 15 or ty < 0 or ty >= 15 or board[tx][ty] != color:
break
count += 1
for j in range(1, 5):
tx = x - j * dx[i]
ty = y - j * dy[i]
if tx < 0 or tx >= 15 or ty < 0 or ty >= 15 or board[tx][ty] != color:
break
count += 1
if count >= 5:
return True
return False
# 下棋并判断胜负
def move(x, y, color):
board[x][y] = color
if can_move(x, y, color):
return True
return False
```
在实际游戏中,还需要考虑如何判断哪方胜利,以及如何进行人机交互等问题。以上代码只是五子棋算法的一个简单示例,具体实现还需要结合实际情况进行调整。
python 判断五子棋 算法代码
以下是一个简单的基于Python语言实现的五子棋判断算法代码示例:
```python
# 定义棋盘大小
BOARD_SIZE = 15
# 定义棋子颜色
EMPTY = 0
BLACK = 1
WHITE = 2
# 定义判断方向
DIRECTIONS = [(0, 1), (1, 0), (1, 1), (1, -1)]
# 判断某个位置是否在棋盘内
def is_valid_location(x, y):
return x >= 0 and x < BOARD_SIZE and y >= 0 and y < BOARD_SIZE
# 判断当前位置是否为空
def is_empty_location(board, x, y):
return board[x][y] == EMPTY
# 判断当前位置是否为黑子
def is_black_location(board, x, y):
return board[x][y] == BLACK
# 判断当前位置是否为白子
def is_white_location(board, x, y):
return board[x][y] == WHITE
# 判断某个位置是否连成五子
def is_five_in_row(board, x, y, color):
for dx, dy in DIRECTIONS:
count = 1
for i in range(1, 5):
nx, ny = x + i * dx, y + i * dy
if is_valid_location(nx, ny) and is_same_color(board, nx, ny, color):
count += 1
else:
break
for i in range(1, 5):
nx, ny = x - i * dx, y - i * dy
if is_valid_location(nx, ny) and is_same_color(board, nx, ny, color):
count += 1
else:
break
if count >= 5:
return True
return False
# 判断当前位置与指定颜色是否相同
def is_same_color(board, x, y, color):
if color == BLACK:
return is_black_location(board, x, y)
elif color == WHITE:
return is_white_location(board, x, y)
return False
# 判断当前位置是否可以下棋
def is_valid_move(board, x, y, color):
if not is_valid_location(x, y) or not is_empty_location(board, x, y):
return False
board[x][y] = color
is_win = is_five_in_row(board, x, y, color)
board[x][y] = EMPTY
return is_win
# 五子棋判断算法
def is_game_over(board):
for x in range(BOARD_SIZE):
for y in range(BOARD_SIZE):
if is_valid_move(board, x, y, BLACK):
return False
if is_valid_move(board, x, y, WHITE):
return False
return True
```
这段代码实现了基本的五子棋规则判断功能,可以判断某个位置是否可以下棋、是否连成五子等。
相关推荐
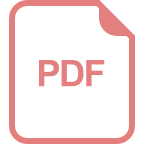
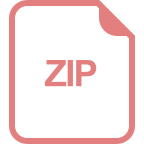
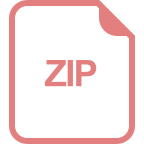












