python判断五子棋算法
时间: 2023-11-10 10:02:41 浏览: 39
五子棋的判断算法可以分为两部分:
1. 水平、垂直和两条对角线方向的判断
2. 以每个点为起点,向四周扫描,判断是否构成五子棋
下面是一个简单的示例代码:
```python
# 棋盘大小
BOARD_SIZE = 15
# 判断水平、垂直和两条对角线方向的算法
def judge(board, x, y, color):
# 判断水平方向
count = 0
for i in range(x - 4, x + 5):
if i < 0 or i >= BOARD_SIZE:
continue
if board[i][y] == color:
count += 1
else:
count = 0
if count == 5:
return True
# 判断垂直方向
count = 0
for i in range(y - 4, y + 5):
if i < 0 or i >= BOARD_SIZE:
continue
if board[x][i] == color:
count += 1
else:
count = 0
if count == 5:
return True
# 判断斜向(左上到右下)
count = 0
for i in range(-4, 5):
if x + i < 0 or x + i >= BOARD_SIZE or y + i < 0 or y + i >= BOARD_SIZE:
continue
if board[x + i][y + i] == color:
count += 1
else:
count = 0
if count == 5:
return True
# 判断斜向(左下到右上)
count = 0
for i in range(-4, 5):
if x + i < 0 or x + i >= BOARD_SIZE or y - i < 0 or y - i >= BOARD_SIZE:
continue
if board[x + i][y - i] == color:
count += 1
else:
count = 0
if count == 5:
return True
return False
# 判断是否构成五子棋
def is_win(board, x, y, color):
if judge(board, x, y, color):
return True
# 判断四个方向
directions = [(0, 1), (1, 0), (1, 1), (-1, 1)]
for dx, dy in directions:
count = 0
for i in range(1, 5):
tx, ty = x + i * dx, y + i * dy
if tx < 0 or tx >= BOARD_SIZE or ty < 0 or ty >= BOARD_SIZE:
break
if board[tx][ty] == color:
count += 1
else:
break
for i in range(1, 5):
tx, ty = x - i * dx, y - i * dy
if tx < 0 or tx >= BOARD_SIZE or ty < 0 or ty >= BOARD_SIZE:
break
if board[tx][ty] == color:
count += 1
else:
break
if count >= 4:
return True
return False
```
其中,`board` 为二维数组,表示棋盘,`x` 和 `y` 表示落子的坐标,`color` 表示棋子的颜色(黑或白)。函数 `judge` 判断水平、垂直和两条对角线方向是否构成五子棋,函数 `is_win` 则在 `judge` 的基础上,向四周扫描判断是否构成五子棋。
相关推荐
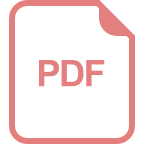
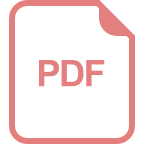
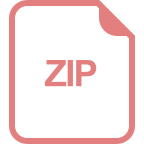














