s->next=g->adjlist[i1].firstedge;
时间: 2024-03-14 17:49:26 浏览: 42
这是一个结构体指针 s 指向的结构体 EdgeNode 中的成员变量 next 被赋值为图 g 中顶点 i1 的邻接表的头指针 firstedge 所指向的结构体。其中 -> 运算符用于访问结构体指针所指向结构体中的成员,相当于 (*s).next = g->adjlist[i1].firstedge; 的简写。通常情况下,顶点的邻接表中的每个结点都有一个指向下一个结点的指针,这个指针就是 next。因此,这条语句的作用是将新创建的结点 s 插入到顶点 i1 的邻接表的头部,使其成为新的邻接点。
相关问题
if(c==0) { s=(EdgeNode *)malloc(sizeof(EdgeNode)); s->adjvex=i1; s->next=g->adjlist[j1].firstedge; g->adjlist[j1].firstedge=s; } }system("cls");
这是一个 if 判断语句。如果变量 c 的值为 0,那么执行 if 语句块中的代码。该代码块首先创建一个新的 EdgeNode 结构体的指针 s,然后将其 adjvex 成员变量赋值为顶点 i1 的编号,将其 next 成员变量赋值为图 g 中顶点 j1 的邻接表的头指针,最后将 s 赋值为顶点 j1 的邻接表的新头结点。这样就将 i1 和 j1 之间的一条边添加到了图 g 中,如果 c 的值不为 0,则不执行该代码块。最后,使用 system 函数清屏。
#include <stdio.h> #include <stdlib.h> #include <string.h> #include <malloc.h> #define MAXV 1000 #define ElemType int #define INF 32767typedef struct { int no; int info; }VertexType; typedef struct{ int edges[MAXV][MAXV]; int n,e; VertexType vexs[MAXV]; }MatGraph; typedef struct ArcNode{ int adjvex; int weight; struct ArcNode *nextarc; }ArcNode; typedef struct VNode{ VertexType data; ArcNode *firstarc; }VNode,AdjList[MAXV]; typedef struct{ AdjList adjlist; int n,e; }AdjGraph; void CreateAdj(AdjGraph *&G,int A [MAXV][MAXV],int n,int e){ int i,j;ArcNode *p; G=(AdjGraph *)malloc(sizeof(AdjGraph)); for(i=0;i<n;i++) { G->adjlist[i].firstarc=NULL; } for(i=0;i<n;i++) { for(j=n-1;j>=0;j--) { if(A[i][j]!=0 && A[i][j]!=INF) { p=(ArcNode *)malloc(sizeof(ArcNode)); p->adjvex=j; p->weight=A[i][j]; p->nextarc=G->adjlist[i].firstarc; G->adjlist[i].firstarc=p; } } } G->n=n;G->e=e; }void DispAdj(AdjGraph *G) { int i;ArcNode *p; for(i=0;i<G->n;i++) { p=G->adjlist[i].firstarc; printf("%3d:",i); while(p!=NULL) { printf("%3d[%d]->",p->adjvex,p->weight); p=p->nextarc; } printf("^\n"); } }typedef struct{ int data[MAXV]; int front,rear; }SqQueue; void InitQueue(SqQueue *&q){ q=(SqQueue *)malloc(sizeof(SqQueue)); q->front=q->rear=-1; } void DestroyQueue(SqQueue *&q){ free(q); } bool QueueEmpty(SqQueue *q){ return q->front == q->rear; } bool enQueue(SqQueue *&q,int e){ if(q->rear ==MAXV -1){ return false; } q->rear++; q->data[q->rear]=e; return true; } bool deQueue(SqQueue *&q,int &e){ if(q->front ==q->rear){ return false; } q->front++; e=q->data[q->front]; return true; }MatGraph *CreateMat(char a[],int n,int e) { MatGraph *G=(MatGraph *)malloc(sizeof(MatGraph)); int i,j,k; G->n=n; G->e=e; for(i=0;i<n;i++) { G->vexs[i].no=i; G->vexs[i].info=a[i]; } for(i=0;i<n;i++) { for(j=0;j<n;i++) { G->edges[i][j]=0; } } for(k=0;k<e;k++) { printf("输入相邻的顶点:"); scanf("%d",&i); G->edges[i][j]=1; G->edges[j][i]=1; } return G; } int main(){ int n=7,e=12; char a[]={'0','1','2','3','4','5','6'}; MatGraph *G=CreateMat(a,n,e); AdjGraph *H; CreateAdj(H,G->edges,n,e); DFS(G,v); return 0; }修改上述代码
在上述代码中需要修改的地方有:
1. 在CreateMat函数中,第二个for循环的条件应该是j<n而不是i<n。
2. 在CreateMat函数中,输入相邻顶点时应该输入j而不是i。
3. 在main函数中,DFS函数的参数应该是G而不是v。
修改后的代码如下:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <malloc.h>
#define MAXV 1000
#define ElemType int
#define INF 32767
typedef struct {
int no;
int info;
} VertexType;
typedef struct{
int edges[MAXV][MAXV];
int n,e;
VertexType vexs[MAXV];
} MatGraph;
typedef struct ArcNode{
int adjvex;
int weight;
struct ArcNode *nextarc;
} ArcNode;
typedef struct VNode{
VertexType data;
ArcNode *firstarc;
} VNode,AdjList[MAXV];
typedef struct{
AdjList adjlist;
int n,e;
} AdjGraph;
void CreateAdj(AdjGraph *&G,int A [MAXV][MAXV],int n,int e){
int i,j;
ArcNode *p;
G=(AdjGraph *)malloc(sizeof(AdjGraph));
for(i=0;i<n;i++)
{
G->adjlist[i].firstarc=NULL;
}
for(i=0;i<n;i++)
{
for(j=n-1;j>=0;j--)
{
if(A[i][j]!=0 && A[i][j]!=INF)
{
p=(ArcNode *)malloc(sizeof(ArcNode));
p->adjvex=j;
p->weight=A[i][j];
p->nextarc=G->adjlist[i].firstarc;
G->adjlist[i].firstarc=p;
}
}
}
G->n=n;
G->e=e;
}
void DispAdj(AdjGraph *G) {
int i;
ArcNode *p;
for(i=0;i<G->n;i++)
{
p=G->adjlist[i].firstarc;
printf("%3d:",i);
while(p!=NULL)
{
printf("%3d[%d]->",p->adjvex,p->weight);
p=p->nextarc;
}
printf("^\n");
}
}
typedef struct{
int data[MAXV];
int front,rear;
} SqQueue;
void InitQueue(SqQueue *&q){
q=(SqQueue *)malloc(sizeof(SqQueue));
q->front=q->rear=-1;
}
void DestroyQueue(SqQueue *&q){
free(q);
}
bool QueueEmpty(SqQueue *q){
return q->front == q->rear;
}
bool enQueue(SqQueue *&q,int e){
if(q->rear ==MAXV -1){
return false;
}
q->rear++;
q->data[q->rear]=e;
return true;
}
bool deQueue(SqQueue *&q,int &e){
if(q->front ==q->rear){
return false;
}
q->front++;
e=q->data[q->front];
return true;
}
void DFS(MatGraph *G,int v){
int i;
printf("%d ",v);
visited[v]=1;
for(i=0;i<G->n;i++){
if(G->edges[v][i]!=0 && !visited[i]){
DFS(G,i);
}
}
}
MatGraph *CreateMat(char a[],int n,int e) {
MatGraph *G=(MatGraph *)malloc(sizeof(MatGraph));
int i,j,k;
G->n=n;
G->e=e;
for(i=0;i<n;i++)
{
G->vexs[i].no=i;
G->vexs[i].info=a[i];
}
for(i=0;i<n;i++)
{
for(j=0;j<n;j++)
{
G->edges[i][j]=0;
}
}
for(k=0;k<e;k++)
{
int i,j;
printf("输入相邻的顶点:");
scanf("%d %d",&i,&j);
G->edges[i][j]=1;
G->edges[j][i]=1;
}
return G;
}
int main(){
int n=7,e=12;
char a[]={'0','1','2','3','4','5','6'};
MatGraph *G=CreateMat(a,n,e);
AdjGraph *H;
CreateAdj(H,G->edges,n,e);
DFS(G,0);
return 0;
}
```
阅读全文
相关推荐
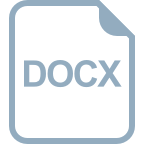
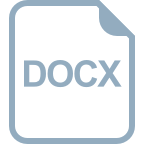













