编写一个C#程序,定义一个接口IShape,该接口包含一个计算面积的方法Area. 然后定义两个类Circle和Rectangle来实现该接口,并分别实现Area方法来计算圆形和矩形的面积。最后,在主程序中创建这两个类的对象并计算它们的面积。
时间: 2024-11-09 18:29:38 浏览: 16
在C#中,首先你需要定义一个名为IShape的接口,它有一个抽象方法`Area()`:
```csharp
public interface IShape
{
double Area();
}
```
接下来,我们创建两个类Circle和Rectangle,它们都实现了IShape接口,并重写了Area()方法:
```csharp
public class Circle : IShape
{
public double Radius { get; set; }
public Circle(double radius)
{
Radius = radius;
}
public double Area()
{
return Math.PI * Radius * Radius;
}
}
public class Rectangle : IShape
{
public double Width { get; set; }
public double Height { get; set; }
public Rectangle(double width, double height)
{
Width = width;
Height = height;
}
public double Area()
{
return Width * Height;
}
}
```
在主程序Main函数中,你可以实例化Circle和Rectangle对象并调用各自的Area()方法:
```csharp
static void Main(string[] args)
{
// 创建Circle对象
IShape circle = new Circle(5);
Console.WriteLine($"Circle area: {circle.Area()}");
// 创建Rectangle对象
IShape rectangle = new Rectangle(4, 6);
Console.WriteLine($"Rectangle area: {rectangle.Area()}");
// 注意这里不需要显式转换,因为它们都是IShape的实例
// 如果需要强制转换,可以写成 (Circle)circle 或 (Rectangle)rectangle
}
```
阅读全文
相关推荐
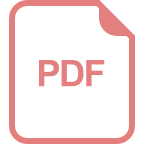
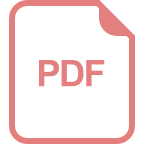
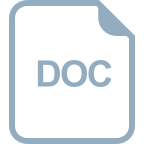




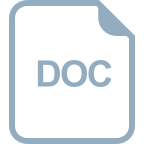
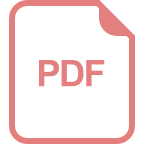
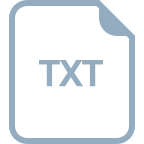
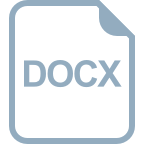
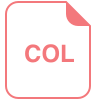
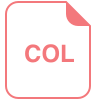
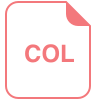
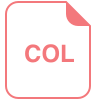



