编写一个c#程序,定义一个接口IShape,该接口包含一个计算面积的方法Area。然后定义两个类Circle和Rectangle来实现该接口,并分别实现Area方法来计算圆形和矩形的面积。最后,在主程序中创建这两个类的对象并计算它们的面积
时间: 2024-11-09 20:31:13 浏览: 38
在C#中,你可以按照以下步骤编写一个程序:
1. 首先,定义一个名为`IShape`的接口,它包含一个计算面积的方法`Area`:
```csharp
public interface IShape
{
double Area();
}
```
2. 然后,创建两个实现了`IShape`接口的类,`Circle`和`Rectangle`,每个类都有自己的`Area`实现:
```csharp
public class Circle : IShape
{
private double radius;
public Circle(double radius)
{
this.radius = radius;
}
public double Area()
{
return Math.PI * radius * radius;
}
}
public class Rectangle : IShape
{
private double width;
private double height;
public Rectangle(double width, double height)
{
this.width = width;
this.height = height;
}
public double Area()
{
return width * height;
}
}
```
3. 在主程序`Main`中,实例化`Circle`和`Rectangle`对象,并调用它们的`Area`方法:
```csharp
static void Main(string[] args)
{
// 创建圆对象并计算面积
IShape circle = new Circle(5);
Console.WriteLine($"Circle area: {circle.Area()}");
// 创建矩形对象并计算面积
IShape rectangle = new Rectangle(4, 6);
Console.WriteLine($"Rectangle area: {rectangle.Area()}");
// 结束程序
Console.ReadLine();
}
```
当你运行这个程序时,它会打印出圆和矩形各自的面积。
阅读全文
相关推荐
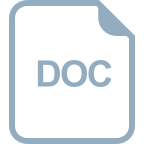
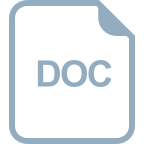
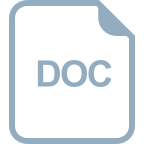




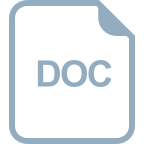
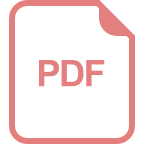
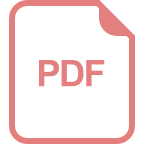
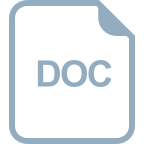
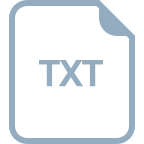
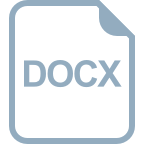
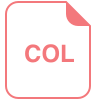
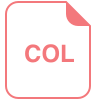
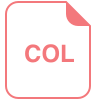
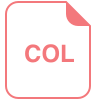

