编写一个C#程序,定义一个接口IShape,该接口包含一个计算面积的方法Area。然后定义两个类Circle和Rectangle来实现该接口,并分别实现Area方法来计算圆形和矩形的面积。最后,在主程序中,创建这两个类的对象,并计算他们的面积
时间: 2024-11-05 10:28:25 浏览: 10
在C#中,首先你需要定义一个接口`IShape`,用于表示可以计算面积的形状。接着,你会创建两个实现了这个接口的类`Circle`和`Rectangle`,每个类都提供各自的面积计算方法。以下是简单的示例:
```csharp
// 定义接口 IShape
public interface IShape
{
double CalculateArea();
}
// 圆形类 Circle 实现 IShape 接口
public class Circle : IShape
{
public double Radius { get; set; }
// Implement the CalculateArea method for circles
public double CalculateArea()
{
return Math.PI * Radius * Radius;
}
}
// 矩形类 Rectangle 也实现 IShape 接口
public class Rectangle : IShape
{
public double Width { get; set; }
public double Height { get; set; }
// Implement the CalculateArea method for rectangles
public double CalculateArea()
{
return Width * Height;
}
}
// 主程序 Main
using System;
class Program
{
static void Main(string[] args)
{
// 创建 Circle 和 Rectangle 对象并计算它们的面积
IShape circle = new Circle() { Radius = 5 };
IShape rectangle = new Rectangle() { Width = 10, Height = 8 };
// 计算并打印面积
Console.WriteLine($"Circle area: {circle.CalculateArea()}");
Console.WriteLine($"Rectangle area: {rectangle.CalculateArea()}");
// 结束程序
Console.ReadLine();
}
}
```
在这个例子中,尽管`Circle`和`Rectangle`有不同的内部计算方式,但通过`IShape`接口,你可以统一处理所有支持计算面积的对象。
阅读全文
相关推荐
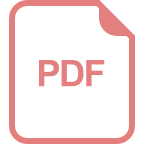
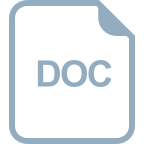
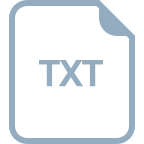




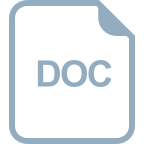
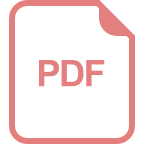
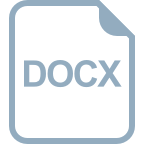
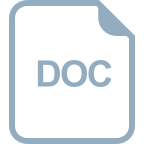
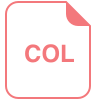
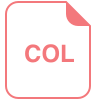
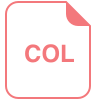
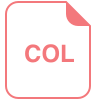



