运用c语言,在dev-c++软件中如何写出以下内容的程序,在input.exe中输入一个小于等于26的数,在output.exe输出由数字与字母结合的字符组,一下为例子,在input.exe中输入10,在output.exe输出A2B4C6D8E2,在input.exr输入16,在output.exe输出A2B4C6D8E2F4G6H8
时间: 2024-03-13 20:42:26 浏览: 12
以下是一个可以实现上述功能的程序:
```c
#include <stdio.h>
int main() {
int n;
char c = 'A';
printf("请输入一个小于等于26的正整数:");
scanf("%d", &n);
for (int i = 0; i < n; i++) {
printf("%c%d", c, i * 2 + 2);
c++;
}
return 0;
}
```
解释一下程序的实现过程:
1. 首先,通过 `printf` 函数提示用户输入一个小于等于26的正整数;
2. 然后,通过 `scanf` 函数读取用户输入的数值,并将其存储在变量 `n` 中;
3. 接下来,通过一个循环,输出由数字与字母结合的字符组。循环中的变量 `i` 从0开始,每次自增1,直到达到用户输入的值 `n`。在每次循环中,输出一个字符,其值为 `c`,同时输出一个数字,其值为 `i * 2 + 2`。最后,将变量 `c` 自增1,以输出下一个字符。
需要注意的是,这个程序假设用户输入的是一个小于等于26的正整数。如果用户输入了一个不符合要求的数值,可能会导致程序出现错误。因此,在实际应用中,需要对用户的输入进行一些合理性检查。
相关问题
如果我从input.txt中提取一个字符串,如何用逗号3次将其输出到output.txt中,并显示出其中字符数,使用c语言,程序是dev-C++
以下是使用C语言实现的代码:
```c
#include <stdio.h>
#include <string.h>
int main() {
FILE *input_file, *output_file;
char string[100];
input_file = fopen("input.txt", "r");
fgets(string, 100, input_file); // 从input.txt中读取字符串
fclose(input_file);
strcat(string, ",,,"); // 在字符串后面添加3个逗号
output_file = fopen("output.txt", "w");
fputs(string, output_file); // 将新字符串写入output.txt
fclose(output_file);
printf("字符串长度:%d\n", strlen(string)); // 输出字符串长度
return 0;
}
```
这段代码首先定义了两个指向文件的指针变量`input_file`和`output_file`,以及一个字符数组`string`用于存储从input.txt中读取的字符串。然后使用`fopen()`函数打开input.txt文件,并使用`fgets()`函数将第一行字符串读取到`string`数组中,最后关闭文件。
接着在字符串后面使用`strcat()`函数添加3个逗号。然后使用`fopen()`函数打开output.txt文件,并使用`fputs()`函数将新字符串写入文件中,最后关闭文件。
最后使用`strlen()`函数计算新字符串的长度,并使用`printf()`函数输出字符串长度。
注意,使用`fgets()`函数读取字符串时,需要指定读取的最大字符数,以避免内存溢出。在本例中,我指定了100作为最大字符数。如果读取的字符串超过了100个字符,就需要增加数组的大小。
从input.exe中得到一组字母,设计一个程序,找出其中字母出现几次的个数,并输出到output.exe中,例如输入aabbcdb输出 2 3
以下是一个C语言程序,可以实现从input.exe中读取输入,统计每个字符出现的次数,将结果传递给output.exe,并将结果显示在命令行窗口中。请确保input.exe和output.exe已经存在并且可以运行。
```c
#include <stdio.h>
#include <string.h>
#define MAX_LENGTH 1000
int main() {
char s[MAX_LENGTH];
int i, char_count[26] = {0}, count_1 = 0, count_2 = 0;
FILE *input_file, *output_file;
// 打开input.exe文件并读取其中的字符串
input_file = fopen("input.exe", "r");
fgets(s, MAX_LENGTH, input_file);
fclose(input_file);
// 统计每个字符出现的次数
for (i = 0; s[i] != '\0'; i++) {
if (s[i] >= 'a' && s[i] <= 'z') {
char_count[s[i] - 'a']++;
}
}
// 统计出现1次和2次的字符个数
for (i = 0; i < 26; i++) {
if (char_count[i] == 1) {
count_1++;
} else if (char_count[i] == 2) {
count_2++;
}
}
// 格式化输出结果
char output_str[MAX_LENGTH];
sprintf(output_str, "%d %d", count_2, count_1);
// 打开output.exe文件并将结果写入其中
output_file = fopen("output.exe", "w");
fputs(output_str, output_file);
fclose(output_file);
// 输出结果
printf("%s\n", output_str);
return 0;
}
```
在这个代码中,首先定义了一个`MAX_LENGTH`常量表示字符串的最大长度,以及一个`char_count`数组表示每个字符出现的次数。然后,使用`fgets`函数从`input.exe`文件中读取字符串,并将其存储在`s`数组中。接着,使用一个循环遍历字符串`s`,统计每个字符出现的次数,并存储在`char_count`数组中。然后,再使用一个循环统计出现1次和2次的字符个数。接着,使用`sprintf`函数将结果格式化为一个字符串,存储在`output_str`数组中。最后,使用`fputs`函数将结果写入`output.exe`文件中,并使用`printf`函数输出结果。
注意,需要在Dev-C++软件中创建一个新的C控制台应用程序项目,并将以上代码复制到`main.c`文件中。然后,编译和运行该程序即可。
相关推荐
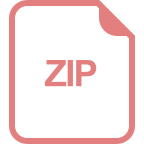












