使用matplotlib绘制动态折线图
时间: 2024-01-08 16:04:01 浏览: 25
以下是使用matplotlib绘制动态折线图的示例代码:
```python
import matplotlib.pyplot as plt
import numpy as np
# 创建一个空的图形对象
fig, ax = plt.subplots()
# 创建一个空的线对象
line, = ax.plot([], [])
# 设置x轴和y轴的范围
ax.set_xlim(0, 10)
ax.set_ylim(0, 20)
# 定义一个更新函数,用于更新折线图的数据
def update(i):
x = np.linspace(0, 10, 100)
y = np.sin(x + i * 0.1)
line.set_data(x, y)
return line,
# 创建一个动画对象,每隔50毫秒调用一次更新函数
ani = animation.FuncAnimation(fig, update, frames=100, interval=50)
# 显示动画
plt.show()
```
这段代码会创建一个空的图形对象和线对象,并设置x轴和y轴的范围。然后定义一个更新函数,该函数会根据参数i的变化更新折线图的数据。最后,使用`animation.FuncAnimation`函数创建一个动画对象,并设置每隔50毫秒调用一次更新函数。最后,调用`plt.show()`显示动画。
相关问题
python使用matplotlib绘制动态折线图
使用matplotlib绘制动态折线图的一般步骤如下:
1. 导入所需的库和模块:
```python
import matplotlib.pyplot as plt
import matplotlib.animation as animation
```
2. 创建一个图形窗口和一个子图:
```python
fig, ax = plt.subplots()
```
3. 定义一个空的数据列表,用于存储动态更新的数据:
```python
data = []
```
4. 定义一个更新数据的函数,用于在每一帧中更新数据:
```python
def update_data(frame):
# 更新数据的逻辑
# 将新的数据添加到data列表中
data.append(new_data)
```
5. 创建一个动画对象,指定更新数据的函数和帧数:
```python
ani = animation.FuncAnimation(fig, update_data, frames=range(num_frames))
```
6. 设置图线格式和其他绘图属性:
```python
# 设置x轴和y轴的范围
ax.set_xlim(0, max_x)
ax.set_ylim(0, max_y)
# 绘制折线图
line, = ax.plot([], [], 'b')
# 设置图例、标题等其他属性
ax.legend(['Line'])
ax.set_title('Dynamic Line Chart')
```
7. 显示动态折线图:
```python
plt.show()
```
请注意,以上步骤仅为一般步骤,具体的实现方式可能会根据具体需求有所不同。
编写matplotlib 绘制动态折线图程序
好的,我可以回答这个问题。编写matplotlib 绘制动态折线图程序的代码如下:
```
import matplotlib.pyplot as plt
import numpy as np
# 创建一个空的折线图
fig, ax = plt.subplots()
line, = ax.plot([], [], lw=2)
# 设置x轴和y轴的范围
ax.set_xlim(, 10)
ax.set_ylim(-1, 1)
# 生成x轴的数据
x = np.linspace(, 10, 100)
# 定义一个更新函数,用于更新折线图的数据
def update(num):
y = np.sin(x + num / 10.)
line.set_data(x, y)
return line,
# 创建动画
from matplotlib.animation import FuncAnimation
ani = FuncAnimation(fig, update, frames=100, interval=50, blit=True)
# 显示折线图
plt.show()
```
这段代码可以生成一个动态的折线图,其中折线的形状会随着时间的推移而变化。
相关推荐
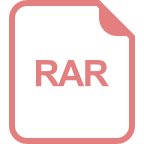
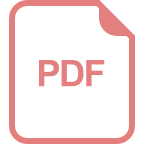
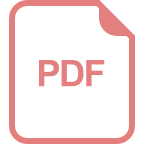











